In this guide, you will learn how to convert an int to string in Java. We can convert int to String using String.valueOf() or Integer.toString() method. We can also use String.format() method for the conversion.
1. Convert int to String using String.valueOf()
String.valueOf(int i) method takes integer value as an argument and returns a string representing the int argument.
Method signature:
public static String valueOf(int i)
Parameters:
i – integer that needs to be converted to a string
Returns:
A string representing the integer argument
Let’s see the java program:
public class JavaExample { public static void main(String args[]) { int ivar = 111; String str = String.valueOf(ivar); System.out.println("String is: "+str); //output is: 555111 because the str is a string //and the + would concatenate the 555 and str System.out.println(555+str); } }
Output:
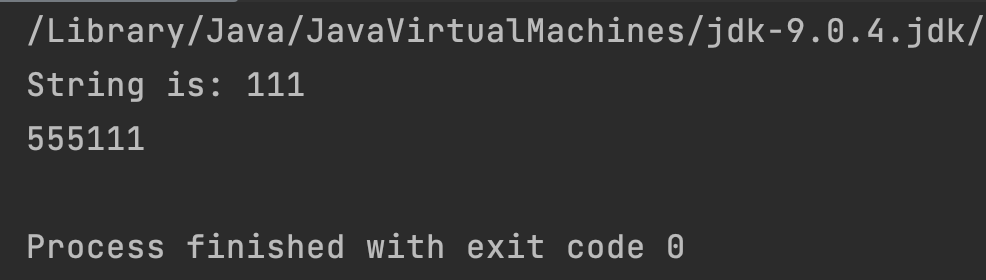
2. Convert int to String using Integer.toString()
Integer.toString(int i) method works same as String.valueOf(int i) method. It belongs to the Integer class and converts the specified integer value to String. for e.g. if passed value is 101 then the returned string value would be “101”.
Method signature:
public static String toString(int i)
Parameters:
i – integer that requires conversion
Returns:
String representing the integer i.
Example:
int ivar2 = 200; String str2 = Integer.toString(ivar2);
Let’s see the complete java program:
public class JavaExample { public static void main(String args[]) { int ivar = 111; String str = Integer.toString(ivar); System.out.println("String is: "+str); //output is: 555111 because the str is a string //and the + would concatenate the 555 and str System.out.println(555+str); //output is: 666 because ivar is int value and the //+ would perform the addition of 555 and ivar System.out.println(555+ivar); } }
Output:
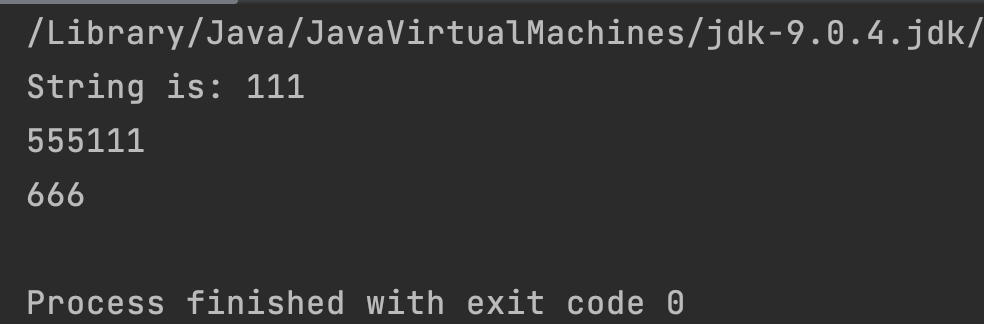
3. Convert int to String using String.format() method
public class JavaExample{ public static void main(String args[]){ int num = 99; String str = String.format("%d",num); System.out.println("hello"+str); } }
Output:
hello99
We can also use format() method to add leading zeroes to the String. Let’s take an example where we are convert int to String with leading zeroes.
Here, %05d
means:
- 0 means pad the String with leading zeroes.
- 5 means set the width of the output string to 5.
public class JavaExample{ public static void main(String args[]){ int num = 15; //leading zeroes to make the string length to 5 String str = String.format("%05d",num); //leading zeroes to make the string length to 3 String str2 = String.format("%03d",num); System.out.println(str); System.out.println(str2); } }
Output:
00015 015
steve says
This is very well presented instruction. One possible addition would be adding explanation of why one might want to do (the example). IE why would I want to convert a integer to a string? Probably a simple reason but none come to mind besides I could do it.