The MonthDay class represents the date as the combination of Month and Day such as --11-25
. This class only represents the Month and day, it doesn’t represents the year, time or time-zone.
Java MonthDay class:
public final class MonthDay extends Object implements TemporalAccessor, TemporalAdjuster, Comparable<MonthDay>, Serializable
Java MonthDay class – Method Summary
Method | Description |
---|---|
LocalDate atYear(int year) | It combines this month-day with the specified year to create an instance of LocalDate. |
int compareTo(MonthDay other) | It compares this month-day to another month-day and returns an int value. The negative value indicates that this month-day is less than the other month-day and the positive value indicates that this month-day is greater than the other month-day. |
boolean equals(Object obj) | Checks if this month-day is equal to the other specified month-day. |
int getMonthValue() | Gets month value from this month-day. The value ranges from 1 to 12 where 1 represents “January” and 12 represents “December”. |
boolean isAfter(MonthDay other) | Checks if this month-day is after the another month-day. |
boolean isBefore(MonthDay other) | Checks if this month-day is before the another month-day. |
static MonthDay now() | It returns the current month-day from the system in default time-zone. |
MonthDay with(Month month) | It returns the copy of the month-day after altering the month with the specified value. |
MonthDay withDayOfMonth(int dayOfMonth) | It returns the copy of this month-day after altering the day of the month value with the specified value. |
Java MonthDay class Example – now() and of() methods
In the following example, we are seeing the usage of now()
and of()
methods.
import java.time.*; public class JavaExample { public static void main(String[] args) { //now() method : returns current month & day MonthDay monthDay = MonthDay.now(); System.out.println("Current Month & Day: "+monthDay); //of method() : returns month & day with the specified value MonthDay monthDay2 = MonthDay.of(12, 25); System.out.println("Specified Month & Day:"+monthDay2); } }
Output:
Current Month & Day: --06-12 Specified Month & Day:--12-25
Java MonthDay class Example – range() & isValidYear() methods
Here we will learn how to use range()
and isValidYear()
methods. The range()
method is used to find the range of the month & days. The isValidYear()
method checks whether the specified year is valid or not.
import java.time.*; import java.time.temporal.*; public class JavaExample { public static void main(String[] args) { //range() method MonthDay month = MonthDay.now(); ValueRange range1 = month.range(ChronoField.MONTH_OF_YEAR); System.out.println("Month Range: "+range1); ValueRange range2 = month.range(ChronoField.DAY_OF_MONTH); System.out.println("Day range: "+range2); //isValidYear() method boolean flag = month.isValidYear(2022); System.out.println("Is 2022 valid year?: "+flag); } }
Output:
Month Range: 1 - 12 Day range: 1 - 30 Is 2022 valid year?: true
Java MonthDay class – get() method example
The get()
method is used to get the specified field from this month-day. In this example, we fetched the MONTH_OF_YEAR
and DAY_OF_MONTH
value from this month-day.
import java.time.*; import java.time.temporal.*; public class JavaExample{ public static void main(String[] args) { MonthDay monthDay = MonthDay.now(); System.out.println("Current date: "+monthDay); long m = monthDay.get(ChronoField.MONTH_OF_YEAR); System.out.println("Month of year: "+m); long d = monthDay.get(ChronoField.DAY_OF_MONTH); System.out.println("Day of month: "+d); } }
Output:
Current date: --06-12 Month of year: 6 Day of month: 12
The other possible values that you can find using get()
method are:
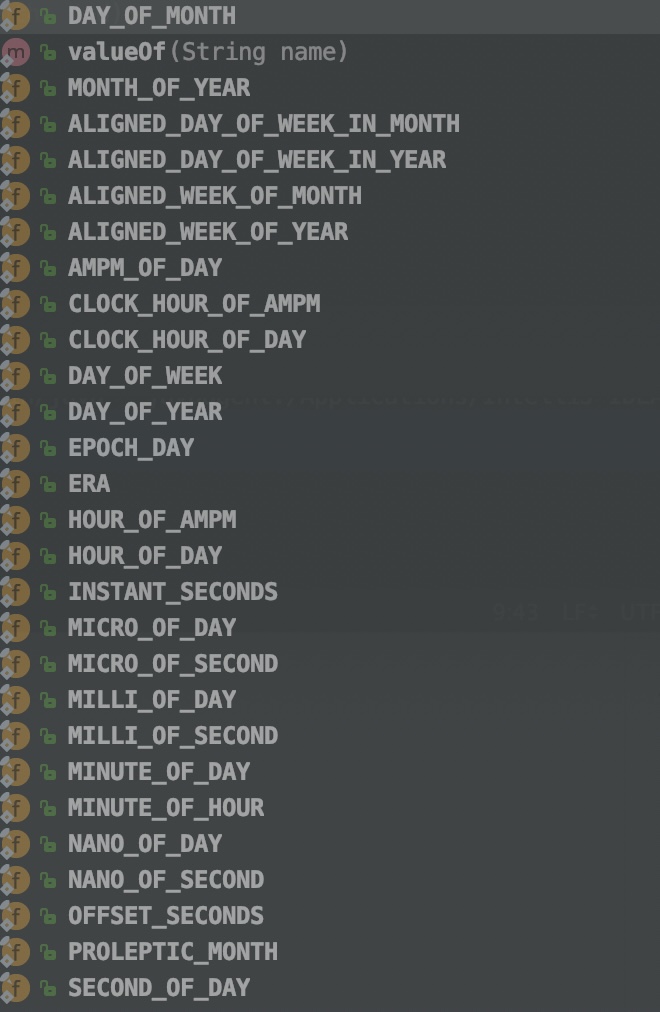
Java MonthDay – isBefore(), isAfter() & withMonth() methods example
Methods isBefore()
and isAfter()
are used for comparison, they just returns true or false based on the comparison. If this month-day is before the specified month-day then isBefore()
will return true else it returns false.
Method withMonth()
alters the month field of this month-day with the specified value. Other with methods are with(Month month)
& withDayOfMonth(int dayOfMonth)
.
import java.time.*; public class JavaExample{ public static void main(String[] args) { MonthDay monthDay = MonthDay.now(); System.out.println("Current date: "+monthDay); MonthDay monthDay2 = monthDay.withMonth(2); System.out.println("Altered Date: "+monthDay2); //Is current date before altered date? System.out.println(monthDay.isBefore(monthDay2)); //Is current date after altered date? System.out.println(monthDay.isAfter(monthDay2)); } }
Output:
Current date: --06-12 Altered Date: --02-12 false true
Leave a Reply