In this tutorial, you will learn how to print Right Pascal’s triangle in Java.
Right Pascal triangle illustration:
number of rows = 5 * * * * * * * * * * * * * * * * * * * * * * * * *
Example 1: Program to print Right Pascal’s Triangle Pattern
In this program, we have hardcoded the number of rows that needs to be printed. To select how many rows you want to print, refer the next example.
public class JavaExample { public static void main(String[] args) { //Initializing number of rows in the pattern //This represents the row with the max stars int numberOfRows= 6; //There are two outer for loops in this program //This is Outer Loop 1: This prints the first half of // the Right Pascal triangle pattern for (int i= 0; i<= numberOfRows-1; i++) { //Prints the stars and whitespaces between them for each row for (int j=0; j<=i; j++) { System.out.print("*"+ " "); } //To move the cursor to new line for next row System.out.println(); } //Outer Loop 2: Prints second half of the triangle for (int i=numberOfRows-1; i>=0; i--) { //Prints the stars and spaces between them for each row //same as the loop in the first half of the pattern for(int j=0; j <= i-1;j++) { System.out.print("*"+ " "); } //Move the cursor to new line after printing a row System.out.println(); } } }
Output:
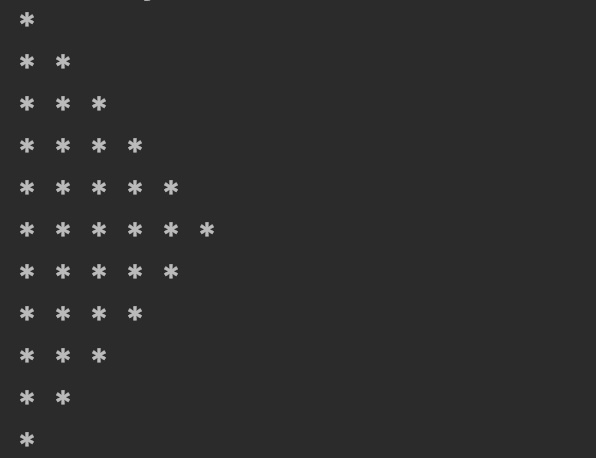
Example 2: Print Right Pascal’s Triangle based on user input
This program is same as above program except that here the number of rows is entered by user.
import java.util.Scanner; public class JavaExample { public static void main(String[] args) { //This represents the number of rows in the pattern int numberOfRows; System.out.print("Enter the number of rows: "); //Getting the number of rows from user Scanner sc = new Scanner(System.in); numberOfRows = sc.nextInt(); //This outer loop prints the first half of the pattern for (int i= 0; i<= numberOfRows-1; i++) { //Prints the stars and in between spaces for each row for (int j=0; j<=i; j++) { System.out.print("*"+ " "); } //new line for next row System.out.println(); } //This outer loop prints the second half of the pattern for (int i=numberOfRows-1; i>=0; i--) { //Prints the stars and in between spaces for each row //in the second half of the pattern for(int j=0; j <= i-1;j++) { System.out.print("*"+ " "); } //new line for next row System.out.println(); } } }
Output:
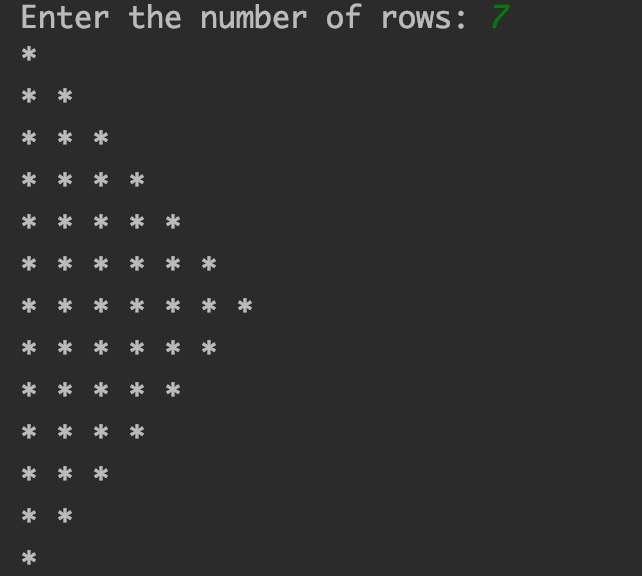
Leave a Reply