In this tutorial, we will write a java program to print the right triangle star pattern.
This is how a right triangle pattern looks like:
Number of rows = 6
Output:
*
* *
* * *
* * * *
* * * * *
* * * * * *
Program to print Right Triangle Star Pattern
This program is fairly easy to understand. We will work with three variables in this program, row
which represents the rows of the pattern, column
that represents the columns of the pattern and numberOfRows
that represents the count of the rows that needs to be printed.
Logic used in the program to print Right Triangle pattern:
Here we are using nested for loop, the outer loop is for rows, which runs from 0 till numberOfRows
(this is 8 in our example), this means that it will print 8 rows of stars.
The inner loop is for column that will print one star in first row, two stars in second row and so on. This is acheived by running inner loop from 0 till column <= row
, this means at the first iteration when the row
is 0 the inner loop will run once, second iteration when the row
value is 1, the inner loop will run twice and will print two stars and so on.
public class JavaExample { public static void main(String args[]) { int row, column, numberOfRows=8; for(row=0; row<numberOfRows; row++) { for(column=0; column<=row; column++) { System.out.print("* "); } //This is just to print the stars in new line after each row System.out.println(); } } }
Output:
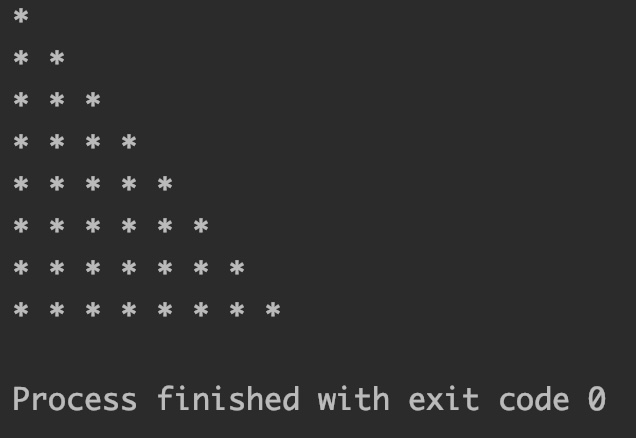
Leave a Reply