The divideUnsigned() method returns the unsigned quotient after dividing first argument by second argument.
Syntax of divideUnsigned() method
public static int divideUnsigned(int dividend, int divisor)
divideUnsigned() Parameters
dividend
– The first int argument. The value to be divided.divisor
– The second int argument. The value that is dividing.
divideUnsigned() Return Value
- Returns unsigned quotient obtained by
dividend / divisor
.
Supported java versions: Java 1.8 and onwards.
Example 1
public class JavaExample { public static void main(String[] args) { int x = 120, y = 20; System.out.println("Unsigned quotient: "+ Integer.divideUnsigned(x, y)); } }
Output:
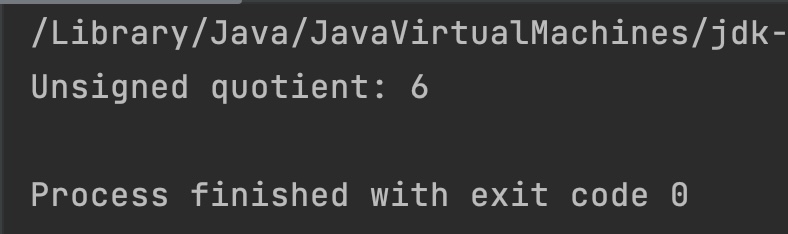
Example 2
public class JavaExample { public static void main(String[] args) { int x = 120, y = -20; //divisor is negative System.out.println("Unsigned quotient: "+ Integer.divideUnsigned(x, y)); } }
Output:

Example 3
The dividend and divisor values are entered by the user.
import java.util.Scanner; public class JavaExample{ public static void main(String[] args){ int x, y; Scanner scan = new Scanner(System.in); System.out.print("Enter first int number: "); x = scan.nextInt(); System.out.print("Enter second int number: "); y = scan.nextInt(); int result = Integer.divideUnsigned(x, y); System.out.println(result); } }
Output:
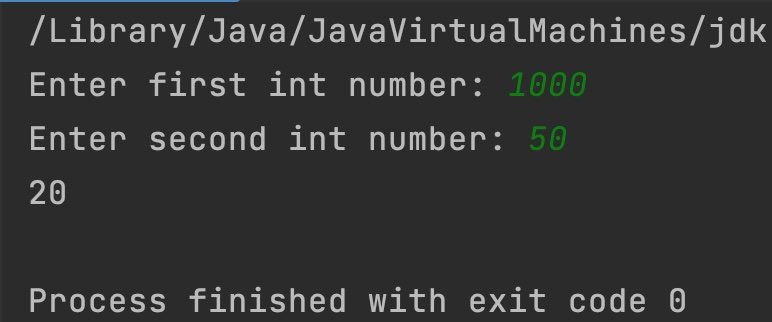