The highestOneBit() method of Integer class, returns an int value with a single one bit in the position of the highest order. This is determined by placing one-bit at the highest position (left most) in the binary representation of a given int number.
For example: If the binary representation of a given number is 0000 0000 0000 0111, then this method returns the int number equivalent to 0000 0000 0000 0100. If the given number is zero, then it returns zero.
Hierarchy:
java.lang Package -> Integer Class -> highestOneBit() Method
Syntax of highestOneBit() method
public static int highestOneBit(int i)
highestOneBit() Parameters
i
– An int value whose highest one bit is to be determined.
highestOneBit() Return Value
- An int value with a single one-bit, placed in the position of highest order one bit.
- Returns zero, if the number itself is zero.
Supported versions: Java 1.5 and onwards.
Example 1
public class JavaExample { public static void main(String[] args) { // 10 binary: 1010 // highest one bit: 1000 == decimal 8 int i = 10; System.out.println(Integer.highestOneBit(i)); } }
Output:
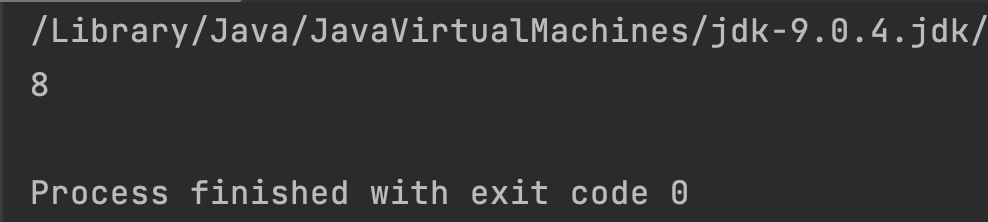
Example 2
public class JavaExample { public static void main(String[] args) { // 31 binary: 11111 // highest one bit: 10000 == decimal 16 int i = 31; System.out.println(Integer.highestOneBit(i)); } }
Output:

Example 3
The highest one bit int value for a negative integer number is equivalent to Integer.MIN_VALUE
.
public class JavaExample { public static void main(String[] args) { int i = -10; System.out.println(Integer.highestOneBit(i)); System.out.println(Integer.MIN_VALUE); } }
Output:
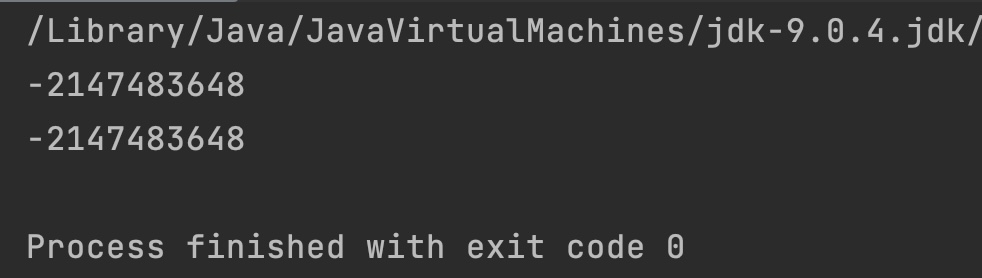