Java Math.random() method returns a random double number between 0.0 and 1.0, where 0.0 is inclusive and 1.0 is exclusive. Number is chosen from this range randomly and each number has equal probability.
public class JavaExample { public static void main(String[] args) { System.out.println(Math.random()); } }
Output: You will most likely get a different output when you run this program.
0.4916566712907685
Syntax of Math.random() method
Math.random(); //returns a random number between 0.0 and 1.0
random() Description
public static double random(): It returns a double number which is greater than equal to 0.0 and less than 1.0.
random() Parameters
- NA
random() Return Value
- Pseudorandom double number from the range of 0.0 and 1.0.
Example 1: Random number between 1 and 100
public class JavaExample { public static void main(String[] args) { double rand = Math.random()*100; System.out.println(rand); } }
Output:
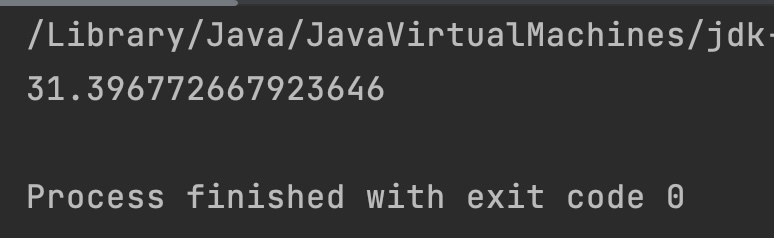
Example 2: Generate negative random number
public class JavaExample { public static void main(String[] args) { double rand = Math.random()*(-10); System.out.println(rand); } }
Output:
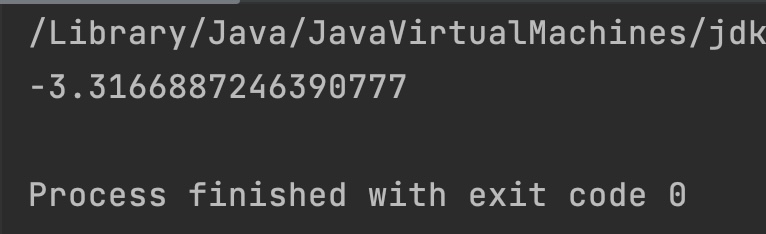
Example 3: Generate random number with no upper limit
public class JavaExample { public static void main(String[] args) { double rand = Math.random()*(Double.MAX_VALUE); System.out.println(rand); } }
Output:
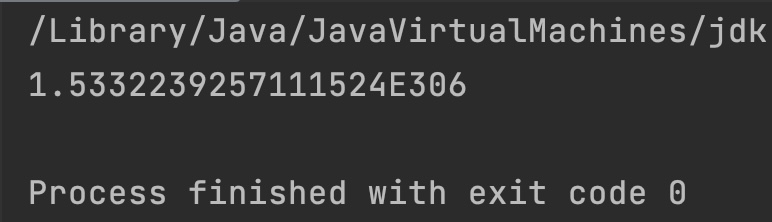