Java StringBuffer indexOf() method returns the first occurrence of the given string in this sequence. In this guide, we will discuss indexOf() method with examples.
Syntax of indexOf() method
sb.indexOf("hello"); //searches string "hello" in the sb sb.indexOf("hello", 4) //starts searching string "hello" from index 4
Here, sb
is an object of StringBuffer
class.
indexOf() Description
There are two variations of indexOf() method in StringBuffer class.
public int indexOf(String str): It returns the index of first occurrence of the string str in the StringBuffer instance.
public int indexOf(String str, int fromIndex): It starts the search from the specified index fromIndex
and returns the index of string str.
indexOf() Parameters
- str: The String str represents the character sequence that needs to be searched in the StringBuffer instance
sb
. - fromIndex: Represents an index from where the search starts.
indexOf() Return Value
- It returns an integer value that represents the index of the first occurrence of specified string. If the specified string is not found then this method returns -1.
Example 1: Find first occurrence of given string
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Cool Book"); System.out.println("Given String: " + sb); // first occurrence of string "oo" System.out.println("Index of string 'oo': "+sb.indexOf("oo")); } }
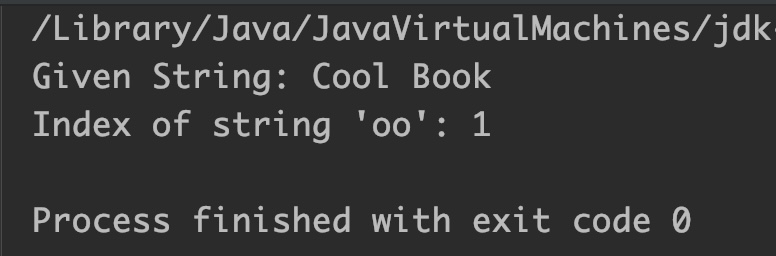
Example 2: Find string after a given index
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Cool Book"); System.out.println("Given String: " + sb); // Search string "oo" after index 3 System.out.println("Occurrence of 'oo' after index 3 is: "+ sb.indexOf("oo", 3)); } }
Output:
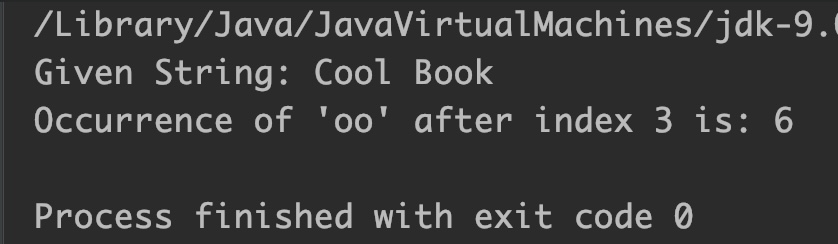
Example 3: Specified string is not found
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Cool Book"); System.out.println("Given String: " + sb); // Index of string "Text" in the given string System.out.println("Index of 'Text' is: "+ sb.indexOf("Text")); } }
Output:
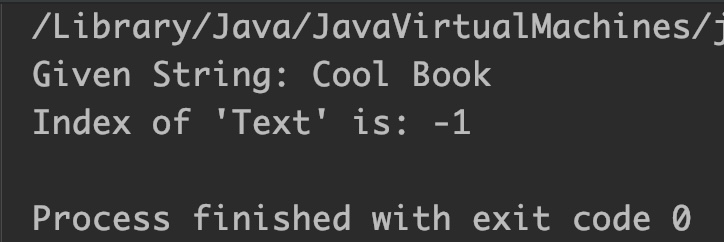
Example 4: Given string is not found after the specified index
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Cool Book"); System.out.println("Given String: " + sb); // Index of string "oo" after index 15. String "oo" // exists in the given sequence but it is not present after // index 15 so the indexOf() method would return -1 System.out.println("Index of 'oo' after 15: "+sb.indexOf("oo",15)); } }
Output:
