Java StringBuffer lastIndexOf() method returns the index of last occurrence of the given string in this sequence. In this tutorial, we will discuss the lastIndexOf() method with examples.
Syntax of lastIndexOf() method
//Returns the index of last occurrence of string "welcome" sb.lastIndexOf("welcome"); //Returns index of last occurrence of "welcome" before index 5 sb.lastIndexOf("welcome", 5);
Here, sb
is an object of StringBuffer class.
lastIndexOf() Description
There are two variations of lastIndexOf() method in Java StringBuffer class.
public int lastIndexOf(String str): It returns the index of last occurrence of the string str in the StringBuffer instance.
public int lastIndexOf(String str, int fromIndex): It returns the index of last occurrence of specified string before the fromIndex
.
lastIndexOf() Parameters
- str: The String str represents the character sequence that needs to be searched in the StringBuffer instance
sb
. - fromIndex: It represents an index. The search of the string str ends here.
lastIndexOf() Return Value
- It returns an integer value that represents the index of the last occurrence of specified string str. If the specified string is not found then this method returns -1.
Example 1: Find last Occurrence of a given string
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Cool Book"); System.out.println("Given String: " + sb); // last occurrence of string "oo" System.out.println("Last Occurrence of String 'oo': "+ sb.lastIndexOf("oo")); } }
Output:
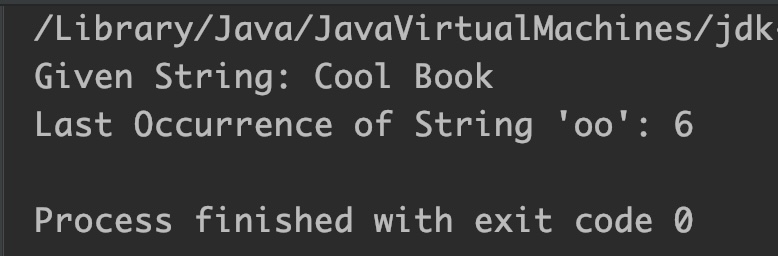
Example 2: Search string before a given index
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("CatBatRat"); System.out.println("String: " + sb); // last occurrence of string "at" before 6 System.out.println("Last Occurrence of 'at' before index 6: "+ sb.lastIndexOf("at", 6)); } }
Output:
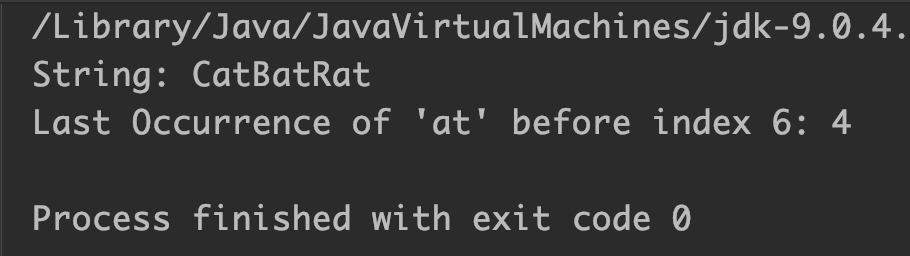
Example 3: If search string is not found
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Cool Book"); System.out.println("String: " + sb); // last occurrence of string "Pen" System.out.println("Last Occurrence of 'Pen': "+ sb.lastIndexOf("Pen")); } }
Output:
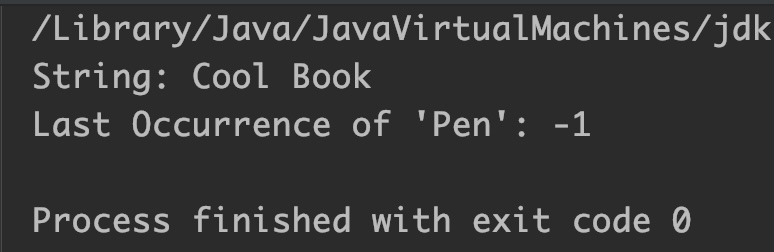
Example 4: If given string is not found before given index
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("My Cat and Rat"); System.out.println("String: " + sb); // last occurrence of string "at" before 3 System.out.println("Last Occurrence of 'at' before index 3: "+ sb.lastIndexOf("at", 3)); } }
Output:
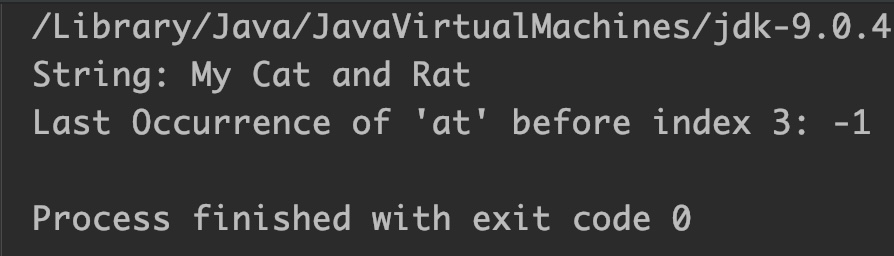