Java StringBuilder setLength() method is used to set a new length to the existing StringBuilder sequence.
If the new length is greater than the current length then null characters are appended at the end of the sequence.
Syntax of setLength() Method:
sb.setLength(5); //set the length of sb to 5
Here, sb represents the object of Java StringBuilder class.
setLength() Description
public void setLength(int newLength): Changes the length of the current StringBuilder instance to the new length.
setLength() Parameters
- newLength: It is an integer value that represents the new length.
setLength() Return Value
- It has void return type as it does not return anything.
- It throws
IndexOutOfBoundsException
, if the specified newLength is a negative number.
Example 1: When new length is less than old length
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("BeginnersBook"); System.out.println("Old String: "+sb); System.out.println("Old length: "+sb.length()); //Set new length for this char sequence sb.setLength(9); System.out.println("New String: "+sb); System.out.println("New length: "+sb.length()); } }
Output:
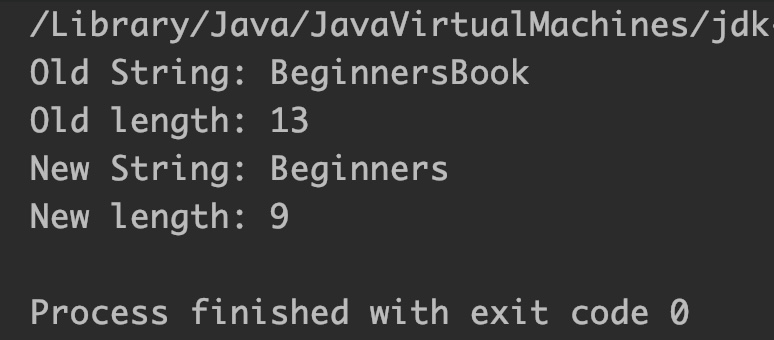
Example 2: When new length is greater than old length
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("BeginnersBook"); System.out.println("Old String: "+sb); System.out.println("Old length: "+sb.length()); //Set new length for this char sequence sb.setLength(20); // 7 null characters are appended at the end System.out.println("New String: "+sb); System.out.println("New length: "+sb.length()); } }
Output:
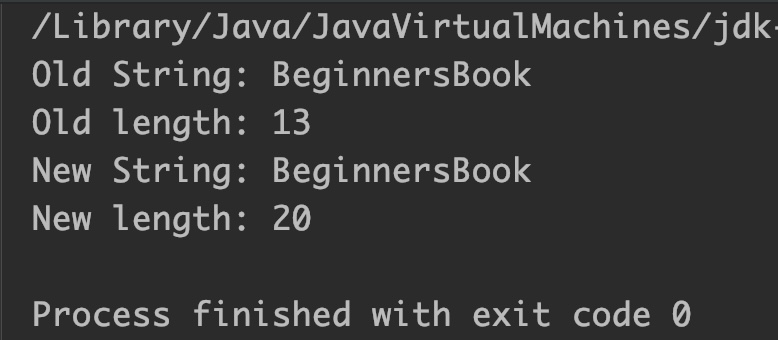
Example 3: When new length is negative
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Hello"); //New length is negative sb.setLength(-2); System.out.println("New String: "+sb); System.out.println("New length: "+sb.length()); } }
Output:
