In this article, we will learn how to write a C program to find sum of array elements. For example, if the array is [1, 2, 3, 4, 5] then the program should print 1+2+3+4+5 = 15 as output. We will see various different ways to accomplish this.
Example 1: Program to find sum of array elements using loops
In this program, we are using for loop to find the sum of elements of array. The explanation of the program is at the end of this code.
#include <stdio.h>
int main()
{
int arr[100],size,sum=0;
printf("Enter size of the array: ");
scanf("%d",&size);
printf("Enter the elements of the array: ");
for(int i=0; i<size; i++)
{
scanf("%d",&arr[i]);
}
//calculating sum of entered array elements
for(int i=0; i<size; i++)
{
sum+=arr[i];
}
printf("Sum of array elements is: %d",sum);
return 0;
}
Explanation of the program:
1. Include Standard Input/Output Library:
#include <stdio.h>
This header file is included so that we can use standard input/output functions such as printf
and scanf
.
2. Main Function:
int main()
This is the main function of the program, the execution of the program begins here.
3. Variable Declarations:
int arr[100], size, sum=0;
arr[100]
: This is to hold array elements entered by user.size
: This is to hold the number of elements user want to enter.sum
: An integer variable initialized to 0, This will hold the sum of all elements.
4. Prompt for Array Size:
printf("Enter size of the array: ");
scanf("%d", &size);
printf
: Prompts the user to enter the size of array.scanf
: Reads user input and stores it intosize
variable.
5. Prompt for Array Elements:
printf("Enter the elements of the array: ");
for(int i=0; i<size; i++)
{
scanf("%d", &arr[i]);
}
printf
: Prompts the user to enter the elements of array.for
loop: It will runsize
(for example, if user enters size as 4, then the loop will run 4 times) number of times.scanf
: Reads user input and store the values in arrayarr[]
.
6. Calculate the Sum of Array Elements:
for(int i=0; i<size; i++)
{
sum += arr[i];
}
for
loop: Iterates over each element of the array (from index 0 tosize-1
).sum += arr[i]
: Adds each element of the array to thesum
variable.
7. Print the result:
printf("Sum of array elements is: %d", sum);
printf
: Prints the sum of the array elements.
8. Return Statement:
return 0;
return 0
: Indicates that the program executed successfully.
Output:
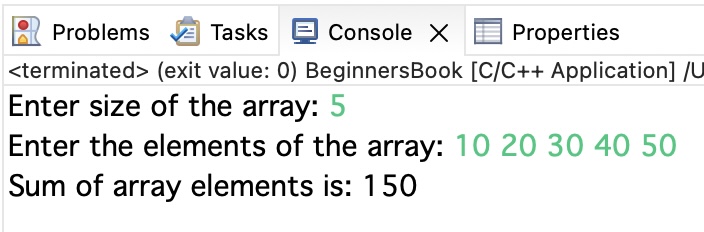
Example 2: Sum of array elements using Recursion
This program calls the user defined function sum_array_elements() and the function calls itself recursively. Here we have hardcoded the array elements but if you want user to input the values, you can use a for loop and scanf function, same way as I did in the next section (Method 2: Using pointers) of this post.
#include <stdio.h>
int sum_array_elements( int arr[], int n ) {
if (n < 0) {
//base case:
return 0;
} else{
//Recursion: calling itself
return arr[n] + sum_array_elements(arr, n-1);
}
}
int main()
{
int array[] = {1,2,3,4,5,6,7};
int sum;
sum = sum_array_elements(array,6);
printf("Sum of array elements is: %d",sum);
return 0;
}
Output:
Sum of array elements is: 28
Example 3: Sum of array elements using pointers
Here we are setting up the pointer to the base address of array and then we are incrementing pointer and using * operator to get & add the values of all the array elements.
#include<stdio.h> int main() { int array[5]; int i, sum=0; int *ptr; printf("\nEnter array elements (5 integer values):"); for(i=0;i<5;i++) scanf("%d",&array[i]); /* array is equal to base address * array = &array[0] */ ptr = array; for(i=0;i<5;i++) { //*ptr refers to the value at address sum = sum + *ptr; ptr++; } printf("\nThe sum is: %d",sum); }
Output:
Enter array elements (5 integer values): 1 2 3 4 5 The sum is: 15
Leave a Reply