This java tutorial would help you learn Java like a pro. I have shared 1000+ tutorials on various topics of Java, including core java and advanced Java concepts along with several Java programming examples to help you understand better.
All the tutorials are provided in a easy to follow systematic manner. It is for everyone, whether you are a college student looking for learning Java programming for free, or a company employee looking for a particular code snippet while building an application in Java, this Java tutorial would definitely be useful for you. Happy learning!
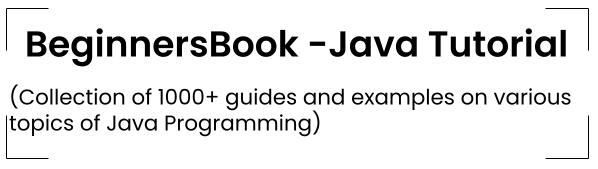
Download Java
Download Java from official website: link
Java Introduction
- Introduction to Java
- History of Java
- Features of Java
- C++ vs Java
- JDK vs JRE vs JVM
- Java Virtual Machine(JVM) Basics
- First Java Program
- Variables in Java
- Java Data Types
- Java TypeCasting
- Java Operators
Java Flow Control
- If-else in Java
- Switch-Case in Java
- Java For loop
- Java While loop
- do-while loop in Java
- Java Continue statement
- Java Break statement
Arrays in Java
Java OOPs
Section 1:
- OOPs Concepts
- Constructor in Java
- Java String
- Java StringBuffer
- Java StringBuilder
- Java Inheritance with example
- Method overloading in Java
- Method overriding in Java
- Method Overloading vs Method Overriding
- Polymorphism in Java
- Aggregation in Java
- Association in Java
Section 2:
- Static keyword in Java
- Super Keyword in Java
- Static and dynamic binding
- Abstract class in Java
- Java Abstract method with example
- Interface in Java
Section 3:
- Java – Abstract class vs interface
- Java Encapsulation with example
- Java Packages with examples
- Access modifiers in Java
- Garbage Collection in Java
- Inner Class
- final keyword
Java Exception Handling
- Java Exception handling
- Java try-catch block
- Java finally block
- How to throw exception in Java
- Java throws keyword
- Custom Exception in Java
- Exception Examples
- Exception propagation
Collections Framework
Section 1:
Section 2:
Section 3:
Section 4:
Java References
Includes collection of guides on various java classes and methods with examples.
Java Programs
Additional Topics
- Java Varargs
- Java Scanner
- Java I/O
- Java Enum
- Java Annotations
- Java Regex
- Java Multithreading
- Java Serialization
- Java AWT Tutorial
- Java Swing Tutorial
- Java autoboxing and unboxing
- Java 8
- Java 9
What is Java?
Java is an object oriented programming language developed by Sun Microsystems in early 1990 by developers James Gosling, Mike Sheridan and Patrick Naughton.
In 1991 James Gosling and his friends formed a team called Green Team to further work on this project. The original idea was to develop this programming language for digital devices such as television, set-top box, remote etc. Later this idea was dropped and Java is developed for internet programming.
Why learn Java?
Java Programming language has several features which other programming languages don’t have. Java receives updates regularly which makes it more robust and future proof. Lots of new features are added on every Java release. There are several features of Java, some of them are:
- Platform independent: Java doesn’t depend on the operating system. For example, a program written on Mac OS can run on Windows and vice versa.
- Easy to learn: Syntax is easy and there are lot of tutorials available online including the one which you are currently reading.
- Performance: Java is significantly faster than other traditional interpreted programming languages. Compiled java code which is known as byte code is like a machine code, that allows a faster execution. Java uses Just in Time compiler which can execute the code on demand, this allows to execute only the method that is being called, which makes it faster and efficient.
Hello World Program
public class JavaExample { public static void main(String[] args){ System.out.println("Hello World"); } }
Official Documentation
- You can also visit the official Oracle website for the java documentation.
Mastanvali says
its very good turorial……….
Subash says
This tutorial is very useful for beginners,I am a tester but learning quickly…………:)
Madhvi says
This is the best tutorial site that teaches Java for free. I am a beginner in Java. I started the java from scratch using this website and learned it in merely 30 days. Highly recommended!
veeresh b says
I am interested in this web site. so kindly help me. I want learn more & I want improve my self.This is best opportunity to improve my subject knowledge.
dinesh yadav says
it’s very useful site for java beginner and we can say awesome and incredible.
Thanks,
Dinesh
Eswar says
superb website for Java Beginners!
Hemant says
you guys should create an app for this website.offline one… there will be lot of downloads
Francis says
The articles in your website that I’ve read so far are very unique and easy to understand. I’m thankful that I found these articles. A great help in time of need.
Imtiaz ahmad says
Hello ! sir its nice tutorials but plz sir upload new tutorials of awt .
Ankit says
Hello Chaitanya,
I am pretty sure that over a period of time you will upload more concepts and tutorials for different technologies, but at the same time I would suggest or better request you to add a frame where you or anybody can post different questions related to particular concept and a person can try writing a code for it. Like a list of programs or something similar to exercises given in Kathy Serra , which on solving can make person better understand the concepts and have hands on programming experience.
Chaitanya Singh says
Seems like a great idea. I would definitely think about it.
Gaurav Chauhan says
Hi buddy,.I have no prior knowledge of any programming language.Can I learn java directly from here and have you covered all java tutorials
Aniruddha says
yes.Its better and easy way …Go ahead..
bisai lakshmana says
This is really helpful for a beginner.It makes us like no need to go for any coaching centers…just simply sit at home and can learn all java topics …..thank you a lot
mathura says
This website is useful for beginners
Gauri says
Hi Chaitanya
Nice tutorials, Can you put them as a series, So that I don’t have to come back to the homepage to view the second tutorial in the list. It is too much back and forth. Also, the series will act as a roadmap for learning java.
Gath says
Hey! Big thanks to the person that thought about making this website. Very helpful for newbie programmers like myself. :)
Dawn Staples says
I am very thankful for this website. You have put together a very good selection of material that is covered in learning Java. A very big thanks to you!
sagar says
Hey! Big thanks to the person that thought about making this website. Very helpful for newbie programmers like myself. :)
steve says
Excellent site. Good job, and thank you. It had been a while since I wrote thread applications and you got me up to speed quickly. Appreciate that. Any input on sockets in java.
Yuliya says
Hi Chaitanya, thanks a lot for such a useful website for those of us who are just starting to learn Java! The basic programs are very helpful.