In this tutorial, you will learn how to generate a random number in Python.
We will use randint()
function of random
module to generate a random number between a given range. To use this function, we must import random
module in our python program.
Syntax of randint() function
random.randint(x,y)
This will generate a random number in the range of x to y, x and y are inclusive in the range, which means the generated random number lies in the range x <= random number <= y
Program to Generate a Random Number in Python
In this program we are generating a random number in the range of 0 to 100 (0 & 100 are inclusive in the range). We are importing the random
module so that we can use the randint()
function, which is defined in the random module.
# Program to generate a random number between 0 and 100 # importing the random module import random print('Generated Random Number:',random.randint(0,100))
Output:
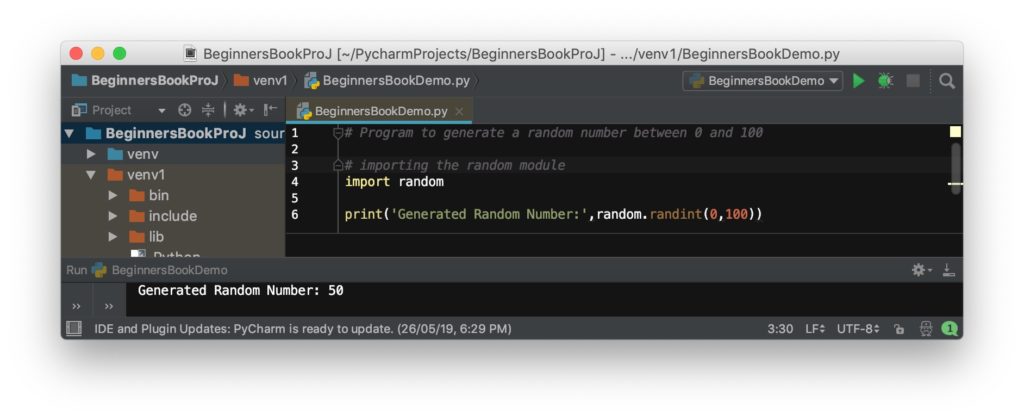
Leave a Reply