In this guide, we will learn how to check if a string is null, empty or blank. First let’s see what is the difference between null, empty or blank string in java.
What is Null String?
A string with no assigned value. For example:
String str = null;
The length of null string is zero and the value is equal to null. To check for null string simply compare the String instance with null, if it is equal to null that means it is a null string. For example:
String myString = null; //null string if(myString==null){ System.out.println("This is a null string"); }
What is an Empty String?
An empty string has a value assigned to it but the length is zero. For example:
String str = ""; //there is no space between quotes
The length of empty string is zero and the value is not equal to null. You can check for empty String like this. The isEmpty() method returns true if the string is empty.
String myString = ""; //empty string if(myString!=null && myString.isEmpty()){ System.out.println("This is an empty string"); }
What is Blank String?
A blank string contains only whitespaces.
String str = " "; //there is a whitespace between quotes
The length of a blank string is not zero, the isEmpty()
method doesn’t return true for blank String. However there is a way to check blank string combining the isEmpty()
method with trim()
method.
Since we know that the blank string contain whitespaces, we can trim the whitespaces using trim()
method and then call isEmpty()
method. This method is shown in the following example.
Example: How to check null, blank and empty String
- Simply compare the string with null to check for null string.
- Use isEmpty() method of string class to check for empty string. The isEmpty() method returns true if the string does not contain any value.
- Use trim() and isEmpty() method together to check for blank string. The trim() method remove all whitespaces and then isEmpty() checks if the string contains any value after removing whitespaces.
public class JavaExample { public static void main(String[] args) { String str1 = null; //null string String str2 = ""; //empty string String str3 = " "; //blank string if(str1==null){ System.out.println("str1 is null string"); }else if(str1.isEmpty()){ System.out.println("str1 is empty string"); }else if(str1.trim().isEmpty()){ System.out.println("str1 is blank string"); } if(str2==null){ System.out.println("str2 is null string"); }else if(str2.isEmpty()){ System.out.println("str2 is empty string"); }else if(str2.trim().isEmpty()){ System.out.println("str2 is blank string"); } if(str3==null){ System.out.println("str3 is null string"); }else if(str3.isEmpty()){ System.out.println("str3 is empty string"); }else if(str3.trim().isEmpty()){ System.out.println("str3 is blank string"); } } }
Output:
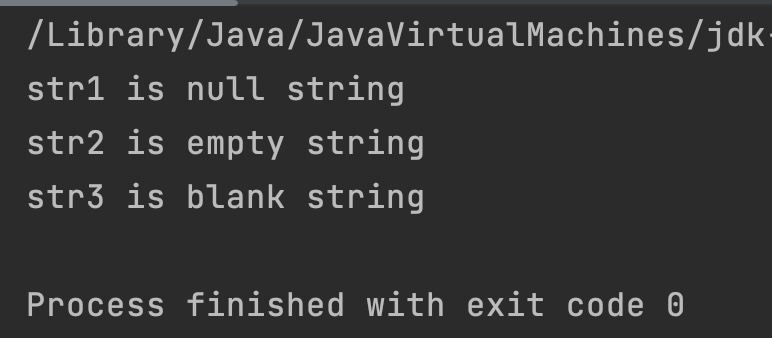