Java StringBuffer codePointCount(int beginIndex, int endIndex) returns the code points count between the given indexes. In this tutorial, we will discuss codePointCount() method with examples.
Syntax of codePointCount() method
int cpCount = sb.codePointCount(3, 6);
This statement will return the code points count for the characters between index 3 and index 6 in this sequence. Here, sb is an object of StringBuffer class.
codePointCount() Description
public int codePointCount(int beginIndex, int endIndex): It looks into the range specified by beginIndex
and endIndex
. Counts the code points available in the range and returns this count.
codePointCount() Parameters
This method takes two parameters:
- beginIndex: Int index, it represents start of the range.
- endIndex: Int Index, it represents end of the range. This index is exclusive so the character present at this index is not included in the code point count.
codePointCount() Return Value
- Returns the code point counts in the specified range.
It throws IndexOutOfBoundsException
, if any of the following condition occurs:
beginIndex < 0
endIndex > sb.length()
beginIndex > endIndex
Example 1: Count code points in a given Sequence
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Hello\uD83D\uDE0A"); //start index first char //end index last char //whole sequence is included in the range int cpCount = sb.codePointCount(0, sb.length()); System.out.println("Sequence is: "+sb); System.out.println("Code Points Count for whole Sequence: "+cpCount); } }
Output:
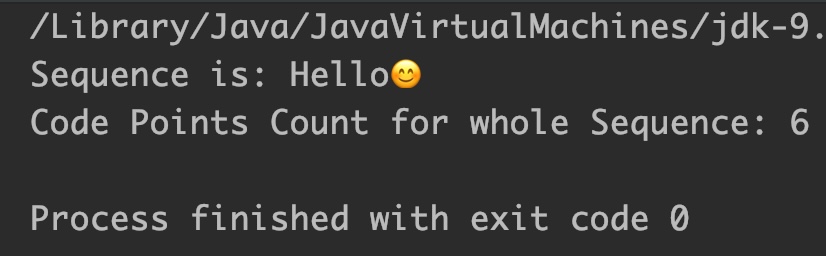
Example 2: Code points count for a subsequence
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Hello\uD83D\uDE0A"); //from index 5 till end int cpCount = sb.codePointCount(5, sb.length()); System.out.println("Sequence is: "+sb); System.out.println("Sub sequence: "+sb.subSequence(5, sb.length())); System.out.println("Code Point Count from index 5 till end: "+cpCount); } }
Output:

Example 3: If beginIndex is greater than endIndex
public class JavaExample { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Hello\uD83D\uDE0A"); //if beginIndex > endIndex int cpCount = sb.codePointCount(3, 2); System.out.println(cpCount); } }
Output:
