The do keyword is used along with while keyword to form a do-while loop.
do{ //body of do-while }while(condition);
Example of do keyword
public class JavaExample { public static void main(String[] args) { int i = 0; do { System.out.println(i); i++; }while (i < 6); } }
Output:
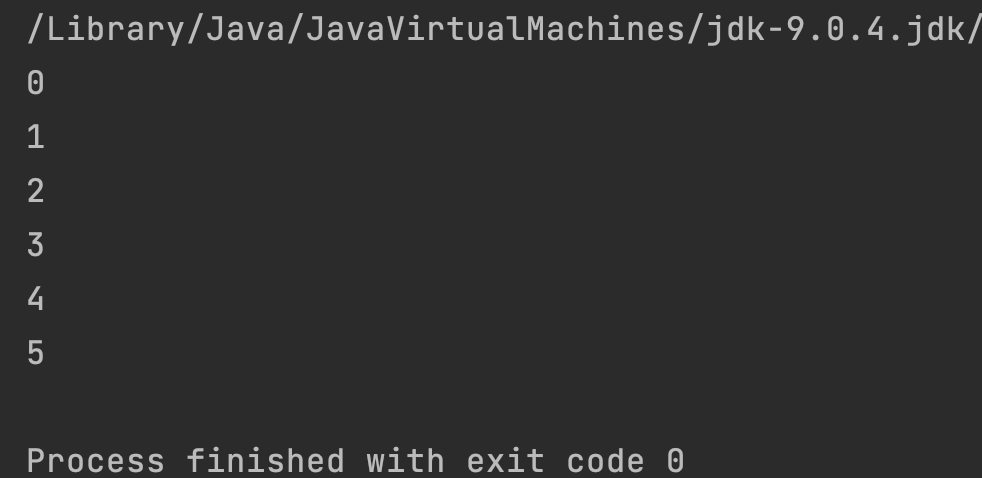
Why we need do while when we have while loop?
The main difference between a while loop and do-while is that:
- while loop checks the condition first and then executes the statements inside it.
- do-while loop executes the statements first and then checks for the condition.
You can say that do-while loop executes at least once for any given condition.
public class JavaExample { public static void main(String[] args) { //do while int i = 0; do { System.out.println("do-while"); i++; }while (i > 1000); //while int j = 0; while (j > 1000){ System.out.println("while"); j++; } } }
Output: As you can see, for the exactly same loop condition and scenario, the while loop didn’t execute but the do-while executed once as it checked the condition after first execution.
do-while