In this guide, we will write a program to split a string by space in Java.
Java Program to split string by space
You can use \\s+
regex inside split string method to split the given string using whitespace. See the following example.
\s
– Matches any white-space character. The extra backslash is to escape the sequence. The + at the end of \\s+
is to capture more than one consecutive spaces between words.
Complete Java code:
public class JavaExample{ public static void main(String args[]){ //a string with space String str = "This is just a text with space"; //split the given string by using space as delimiter String[] strArray = str.split("\\s+"); //prints substrings after split for(String s: strArray){ System.out.println(s); } } }
Output:
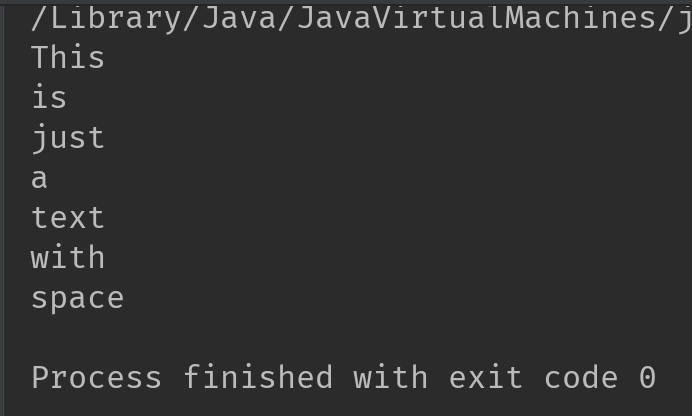
Splitting a string with Multiple Consecutive Spaces
You can also use the following regex inside the split method to split the given string.
str.split("[ ]")
However there is a difference between \\s+
and [ ]
regex.
\\s+
: It consumes all the consecutive spaces and doesn’t produce empty substrings.[ ]
: It splits the string when the first space is encountered, which means if there are multiple consecutive spaces then it would produce empty strings. See the following example:
public class JavaExample{ public static void main(String args[]){ //multiple consecutive whitespaces String str = "Test String"; //split using \\s+ regex String[] strArray = str.split("\\s+"); for(int i=0; i<strArray.length; i++){ System.out.println("strArray["+i+"]"+": "+strArray[i]); } //split using [ ] regex String[] strArray2 = str.split("[ ]"); for(int i=0; i<strArray2.length; i++){ System.out.println("strArray2["+i+"]"+": "+strArray2[i]); } } }
Output:
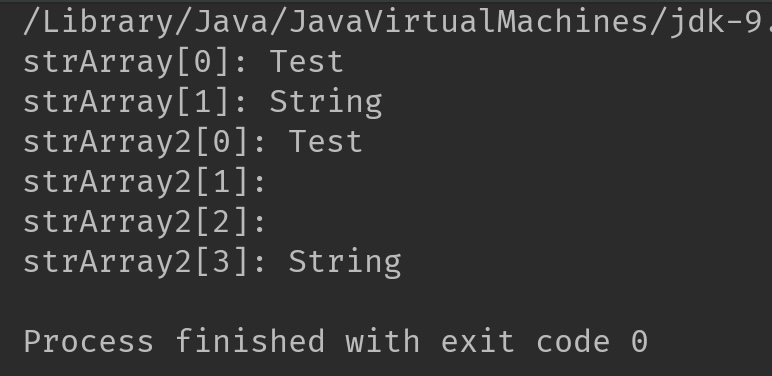
Related guides: