In this tutorial, you will learn how to reverse a number in Java. For example if a given input number is 19
then the output of the program should be 91
. There are several ways to reverse a number in Java. We will mainly discuss following three techniques to reverse a number.
Table of contents
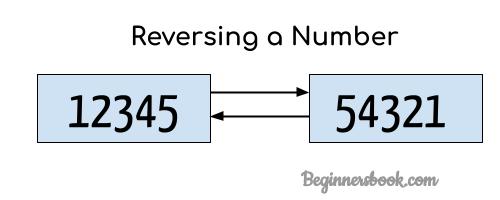
Program 1: Reverse a number using while Loop
- In this program, user is asked to enter a number.
- This input number is read and stored in a variable num using Scanner class.
- The program then uses the while loop to reverse this number.
- Inside the while loop , the given number is divided by 10 using % (modulus) operator and then storing the remainder in the
reversenum
variable after multiplying thereversenum
by 10. When we divide the number by 10, it returns the last digit as remainder. This remainder becomes the first digit ofreversenum
, we are repeating this step again and again until the given number become zero and all the digits are appended in thereversenum
. - At the end of the loop the variable reversenum contains the reverse number and the program prints the value of this variable as output.
import java.util.Scanner; class ReverseNumberWhile { public static void main(String args[]) { int num=0; int reversenum =0; System.out.println("Input your number and press enter: "); //This statement will capture the user input Scanner in = new Scanner(System.in); //Captured input would be stored in number num num = in.nextInt(); //While Loop: Logic to find out the reverse number while( num != 0 ) { reversenum = reversenum * 10; reversenum = reversenum + num%10; num = num/10; } System.out.println("Reverse of input number is: "+reversenum); } }
Output:
Input your number and press enter: 145689 Reverse of input number is: 986541
Program 2: Reverse a number using for Loop
The logic in this program is same as above program, here we are using for loop instead of while loop. As you can see, in this program we have not used the initialization and increment/decrement section of for loop because we have already initialized the variables outside the loop and we are decreasing the value of num
inside for loop by diving it by 10.
import java.util.Scanner; class ForLoopReverseDemo { public static void main(String args[]) { int num=0; int reversenum =0; System.out.println("Input your number and press enter: "); //This statement will capture the user input Scanner in = new Scanner(System.in); //Captured input would be stored in number num num = in.nextInt(); /* for loop: No initialization part as num is already * initialized and no increment/decrement part as logic * num = num/10 already decrements the value of num */ for( ;num != 0; ) { reversenum = reversenum * 10; reversenum = reversenum + num%10; num = num/10; } System.out.println("Reverse of specified number is: "+reversenum); } }
Output:
Input your number and press enter: 56789111 Reverse of specified number is: 11198765
Program 3: Reverse a number using recursion
Here we are using recursion to reverse the number. A method is called recursive method, if it calls itself and this process is called recursion. We have defined a recursive method reverseMethod()
and we are passing the input number to this method.
This method then divides the number by 10, displays the remainder and then calls itself by passing the quotient as parameter. This process goes on and on until the number is in single digit and then it displays the last digit (which is the first digit of the number) and ends the recursion.
Note: You do not get confused between quotient and remainder so let me explain a bit about them here, / operator return quotient and % operator returns remainder. For example: 21/5 would return 4 (quotient), while 21%5 would return 1(quotient).
import java.util.Scanner; class RecursionReverseDemo { //A method for reverse public static void reverseMethod(int number) { if (number < 10) { System.out.println(number); return; } else { System.out.print(number % 10); //Method is calling itself: recursion reverseMethod(number/10); } } public static void main(String args[]) { int num=0; System.out.println("Input your number and press enter: "); Scanner in = new Scanner(System.in); num = in.nextInt(); System.out.print("Reverse of the input number is:"); reverseMethod(num); System.out.println(); } }
Output:
Input your number and press enter: 5678901 Reverse of the input number is:1098765
Example: Reverse an already initialized number
In all the above programs, the number is entered by the user, however if do not want the user interaction part and want to reverse a hardcoded number then this is how you can do it. Here num is initialized with a number, you can just change the value to reverse a different number.
class ReverseNumberDemo { public static void main(String args[]) { int num=123456789; int reversenum =0; while( num != 0 ) { reversenum = reversenum * 10; reversenum = reversenum + num%10; num = num/10; } System.out.println("Reverse of specified number is: "+reversenum); } }
Output:
Reverse of specified number is: 987654321
Related Java Examples :
Sanket says
Reverse number i want explanation mean in layman term ….plz explain the concept of all logic that it happening in middle of program plz explain like u are not writing a program instead your are writing that all math sum in a paper ….
Gabriel says
Hi what does it mean when ” int num=0 “? Does that mean all integers start from 0?
Pankaj says
by int num=0; it says that not garbage velue(a value which affects the outcome of a result) will be stored in the variable num
Sooraj S says
int num=0; means that the variable is initialized with the value zero(0).
Amankumar says
No…here num is a variable and it is assigned as value 0 which mean num has null value so that it can be used further
Kumar says
In Reverse a number using recursion method:
It is not suitable numbers. bez it is failed the test case at
Input as :: 1200 or zero has last digit.
Please correct me if i am wrong.
Thanks
Praveen
Ashwin says
How do i use reverse of a number concept in this palindrome program.. Please help me use it…
Minal says
@ Saket
Possible explanation is:
Let x=123, then ⌊log10x⌋=2. As ⌊x10−1⌋=⌊12.3⌋=12 and ⌊x10−2⌋=⌊1.23⌋=1, we have
123×102−99(12×10+1×1)=123×100−99×121=12300−11979=321
hema says
Hello
can anyone tell me how to reverse a number which starts with zero..like 0213,0334
rufert says
Can someone please help me how to print this in outpu
987654321
87654321
7654321
654321
54321
4321
321
21
1
Liza says
public static void printNumbers(int numbers)
{
String strNum = Integer.toString(numbers);
for (int i = 0; i < strNum.length(); i++)
{
System.out.println(strNum.substring(i));
}
}
public static void main(String[] args)
{
printNumbers(987654321);
}
kiran says
if you want to reverse a number which starts with zero,you should use string data type, as input of 0212 in int data type will takes as 212.
P.Brooks says
How do you add a max string length to the reverse number program? For example, the number must be positive and have a maximum of six digits.
Balaji Singh says
Hi,
I need an help from you .
Question is below :
Consider that you have two ArrayList first ArrayList takes only Strings , and second ArrayList takes only Integer.
Now I want this two Array list to added to HashMap and iterate it also .
So how would you do ?
Balaji Singh says
And Thanks for all your Programs and concepts that you published.
Your concepts are very much good , informative and quite easy to understand.
Avi says
Really great website to start with! ^_^
Kathryn says
Why is it that reversenum isn’t always zero? The way I’m reading, say we input 205. reversenum = 0 * 10
Reversenum= 0 + 5 = 5
num = 205/10 = 20
So printing reversenum would just print 5? I don’t get that.
Issei says
Hello
i am having trouble understanding these lines of code.
Could please explain how it works?
{
reversenum = reversenum * 10;
reversenum = reversenum + num%10;
num = num/10;
}
Sipra Mallick says
Suppose u want to reverse a number 123.
As we have initialized reversenum=0
so,
reversenum = 0 * 10;
reversenum = 0 + 123%10; [123%10=3. so reversenum becomes 3]
num = 123/10;[num stores the value as 12]
In the next iteration,
reversenum = 3* 10;
reversenum = 30 + 12%10;[reversenum=30+2=32.]
num = 12/10;[num stores the value 1]
In the last iteration
reversenum = 32 * 10;
reversenum = 320 + 1%10;[reversenum=320+1=321]
num = 1/10;
Here the looping stops and the result is printed as 321.
Wuu says
executes a block of statements repeatedly until the condition(Boolean expression) returns false, so we choose a number like 325
int num=0;
int reversenum =0;
while( num != 0 )
{
reversenum = reversenum * 10;
reversenum = reversenum + num%10;
num = num/10;
}
the step
1. num =325 != 0
2. reversenum = 0*10=0
3. reversenum = 0+(325%10) = 0+5=5
4. num = 325/10 = 32
5. num =32 !=0
6. reversenum = 5 *10 = 50
7. reversenum = 50 + (32 % 10) = 50+2=52
8. num=32/10 = 3
9 num = 3 != 0
10. reversenum = 52 *10 =520
11. reversenum = 520 + (3 % 10) = 520+3 = 523
12. num = 3/10 =0
final num =0 return false, so we get the reverse number 523.
md raza says
Finally, Now i was able to understand the concept
Ken Santos says
Can anyone write a program that will accept number and produces sequence number in alternate arrangement and reverse order.
Enter number: 5
Output
5142332415
GUL says
why did you multiply reversenum by 10
Sabir says
hello Sir I am facing a problem with the logic.The output of 012 is not coming as 210 only 21! Why?