Java Math.min() method returns the smaller number between two numbers passed as arguments. In this tutorial, we will discuss Math.min() method with examples.
public class JavaExample { public static void main(String args[]) { float f1 = -2.225f; float f2 = -6.5f; //This will print the smaller float number System.out.println(Math.min(f1, f2)); double d1 = 40000; double d2 = 41000; //This will print the smaller double number System.out.println(Math.min(d1, d2)); } }
Output:
-6.5 40000.0
Syntax of Math.min() Method:
Math.min(50, 51) //returns 50
min() Description:
public static int min(int a, int b): Compares the passed integer numbers and returns the smaller integer number. This method has total four variations in the Math class, to compare different data types such as float, long and double. These variations are:
public static int min(int a, int b); public static long min(long a, long b); public static float min(float a, float b); public static double min(double a, double b);
min() Parameters
This method takes two parameters:
- a: The first argument.
- b: The second argument.
min() Return Values
- Returns the smaller number between a and b.
- If one argument is positive and other is negative then min() returns negative argument.
- If the arguments are NaN (Not a Number) then NaN is returned.
- If both the arguments are negative then the argument with higher absolute value is returned. For example: -7 and -3, the min number is -7.
Example 1: Minimum of two integer numbers
public class JavaExample { public static void main(String args[]) { int a = 10, b = 20; int x = -10, y = -20; System.out.println("Minimum of a and b: "+Math.min(a,b)); System.out.println("Minimum of x and y: "+Math.min(x,y)); } }
Output:

Example 2: Minimum of two float numbers
public class JavaExample { public static void main(String args[]) { float a = 12.35f, b = 33.33f; float x = -12.35f, y = -33.33f; System.out.println("Min of a and b: "+Math.min(a,b)); System.out.println("Min of x and y: "+Math.min(x,y)); } }
Output:
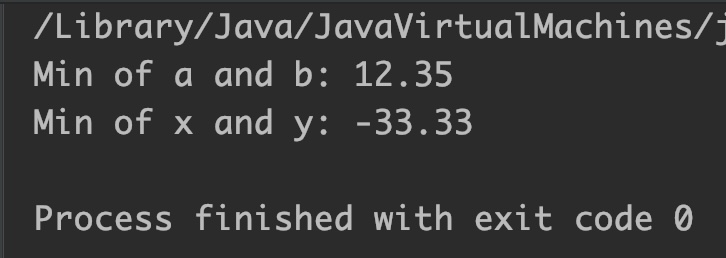
Example 3: Minimum of two double numbers
public class JavaExample { public static void main(String args[]) { double a = 55, b = 56; double x = -55, y = -56; System.out.println("Min of a and b: "+Math.min(a,b)); System.out.println("Min of x and y: "+Math.min(x,y)); } }
Output:
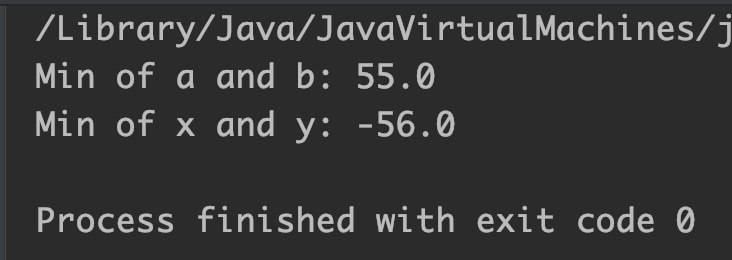
Example 4: Math.min() Method with NaN
public class JavaExample { public static void main(String args[]) { double x =0.0/0; //NaN (Not a Number) System.out.println("Minimum of 10 and NaN: "+Math.min(10, x)); } }
Output:
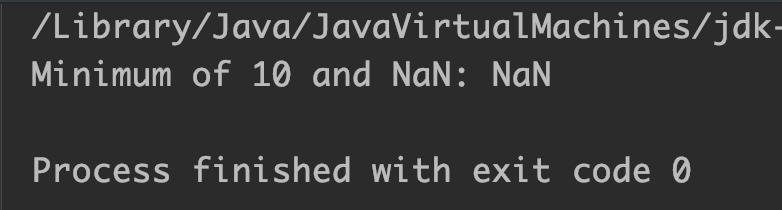