In this tutorial, we will discuss the Java StringBuilder lastIndexOf() method with the help of example programs.
The syntax of lastIndexOf() method is:
//Returns the index of last occurrence of string "welcome" sb.lastIndexOf("welcome"); //Returns index of last occurrence of "welcome" before index 5 sb.lastIndexOf("welcome", 5);
Here, sb
is an object of StringBuilder class.
lastIndexOf() Description
There are two variations of lastIndexOf() method in Java StringBuilder class.
public int lastIndexOf(String str): It returns the index of last occurrence of the string str in the StringBuilder instance.
public int lastIndexOf(String str, int fromIndex): It returns the index of last occurrence of specified string before the fromIndex
.
To get index of first occurrence of specified string use Java StringBuilder indexOf() method.
lastIndexOf() Parameters
The lastIndexOf()
method of Java StringBuilder class takes a single parameter:
- str: The String str represents the character sequence that needs to be searched in the StringBuilder instance
sb
. - fromIndex: It represents an index. The search of the string str ends here.
lastIndexOf() Return Value
- It returns an integer value that represents the index of the last occurrence of specified string str. If the specified string is not found then this method returns -1.
Example 1: Search string without fromIndex
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Text1Text2"); System.out.println("String: " + sb); // last occurrence of string "Text" System.out.println("Last Occurrence of String 'Text': "+ sb.lastIndexOf("Text")); } }
Output:

Example 2: Search string before an index
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("BatRat"); System.out.println("String: " + sb); // last occurrence of string "at" before 3 System.out.println("Last Occurrence of 'at' before index 3: "+ sb.lastIndexOf("at", 3)); } }
Output:
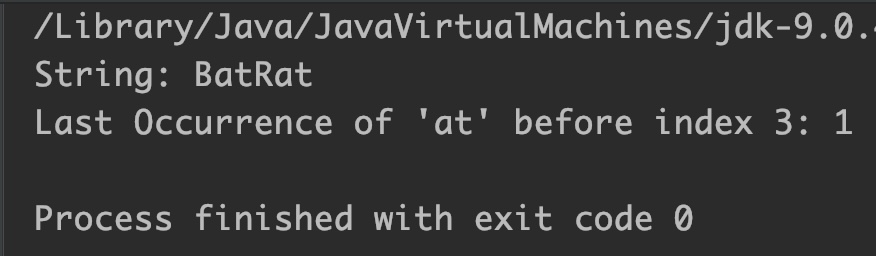
Example 3: When specified string is not found
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("BatRat"); System.out.println("String: " + sb); // last occurrence of string "Pet" System.out.println("Last Occurrence of 'Pet': "+ sb.lastIndexOf("Pet")); } }
Output:

Example 4: When string not found within specified Index
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("BatRat"); System.out.println("String: " + sb); // last occurrence of string "at" before 0 System.out.println("Last Occurrence of 'at' before index 0: "+ sb.lastIndexOf("at", 0)); } }
Output:
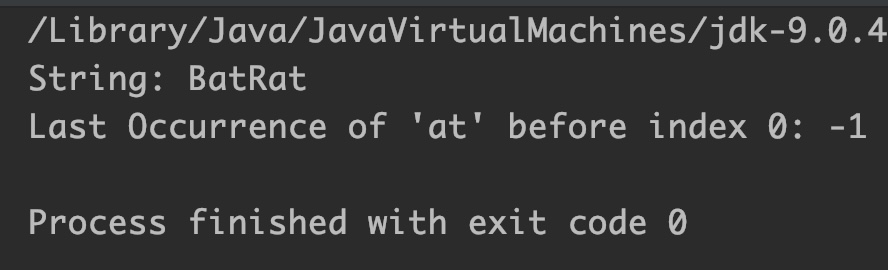