Java Math.ceil() method returns the closest integer value, which is greater than or equal to the given value. For example, Math.ceil(9.9)
would return 10. In this guide, we will discuss the ceil() method with examples.
public class JavaExample { public static void main(String[] args) { double n1 = 5.55, n2 = -5.55; System.out.println(Math.ceil(n1)); System.out.println(Math.ceil(n2)); } }
Output:
6.0 -5.0
Syntax of ceil() method
Math.ceil(9.9); //returns 10
ceil() Description
public static double ceil(double num): Returns a nearest integer value to the passed value num
.
ceil() Parameters
It takes a single parameter:
- num: A double value, whose closest integer to be determined.
ceil() Return Values
- If the given value is an integer, then it returns the same value.
- If the given value is NaN (Not a number) or an infinity or positive zero or negative zero, then the result is the same as the given value.
- If the given value is less than 0 but greater than -1.0, then negative zero is returned.
Example 1: Ceil value for positive and negative number
public class JavaExample { public static void main(String[] args) { double n1 = 19.1, n2 = -19.1; System.out.println(Math.ceil(n1)); System.out.println(Math.ceil(n2)); } }
Output:
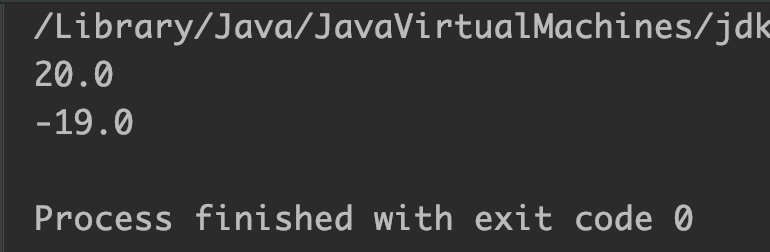
Example 2: Ceil value for a number greater than -1 and less than 0
public class JavaExample { public static void main(String[] args) { // -1 < n < 0 double n = -.15; System.out.println(Math.ceil(n)); } }
Output:

Example 3: Ceil value for positive and negative infinity
public class JavaExample { public static void main(String[] args) { double n1 = 5.0/0; //positive infinity double n2 = -5.0/0; //negative infinity System.out.println(Math.ceil(n1)); System.out.println(Math.ceil(n2)); } }
Output:
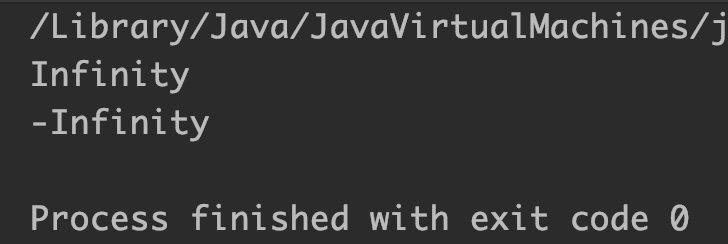
Example 4: Ceil for NaN(Not a Number)
public class JavaExample { public static void main(String[] args) { double n = 0.0/0; //NaN System.out.println("Ceil for NaN: "+Math.ceil(n)); } }
Output:
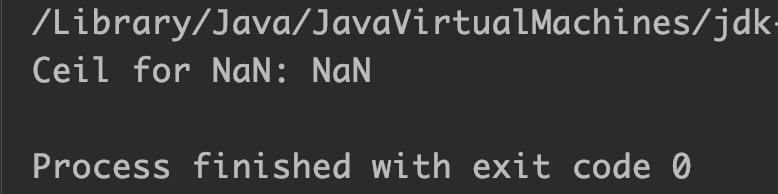