Java Math.cbrt() method returns cube root of a given double value. In this guide, we will discuss cbrt() method with examples.
public class JavaExample { public static void main(String[] args) { double num = 27; System.out.println("Cube root: "+Math.cbrt(num)); } }
Output:
Cube root: 3.0
Syntax of Math.cbrt() Method
Math.cbrt(64); //returns 4.0
cbrt() Description
public static double cbrt(double num): Returns the cube root of the given number num
.
cbrt() Parameters
It takes a single parameter:
- num: A double number whose cube root to be determined.
cbrt() Return Values
- Returns a double value, which is a cube root of the given number.
- If number is NaN (Not a number) then it returns NaN.
- If the given number is negative then it returns the cube root of absolute value with the negative sign.
- If the passed value is infinity, it returns infinity with same sign.
- If the passed value is zero, it returns zero with same sign.
Example 1: Cube root of a given number
public class JavaExample { public static void main(String[] args) { double num = 64; System.out.println("Cube root: "+Math.cbrt(num)); } }
Output:
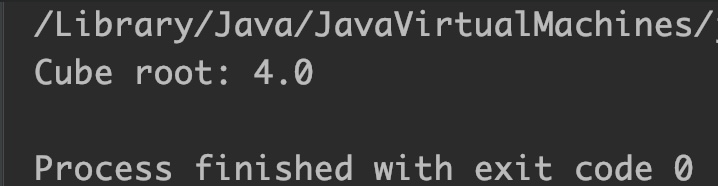
Example 2: Cube root of a negative number
public class JavaExample { public static void main(String[] args) { double num = -125; System.out.println("Cube root of -125: "+Math.cbrt(num)); } }
Output:

Example 3: Cube root of zero, NaN and infinity
public class JavaExample { public static void main(String[] args) { double num = 0; //zero double num2 = 0.0/0; //NaN double num3 = -5.0/0; //negative infinity System.out.println("Cube root of 0: "+Math.cbrt(num)); System.out.println("Cube root of NaN: "+Math.cbrt(num2)); System.out.println("Cube root of -Infinity: "+Math.cbrt(num3)); } }
Output:
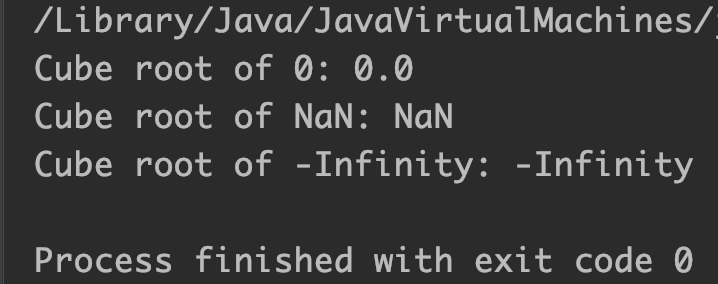