A number which either ends with 7 or divisible by 7 is called Buzz number. For example, 35 is a Buzz number as it is divisible by 7, similarly 47 is also a Buzz number as it ends with 7. In this tutorial, we will write java programs to check Buzz number and print Buzz numbers in a given range.
Program to Check Buzz Number
In this program, we have declared a method checkBuzzNumber()
, which checks whether the number passed as an argument is Buzz or not.
- Condition
num % 10 == 7
checks if the number ends with 7 - Condition
num % 7
checks if the number is divisible by 7 - We have joined these conditions using OR “||” Operator.
import java.util.Scanner; class JavaExample { // This method returns true if num is a Buzz number static boolean checkBuzzNumber(int num) { // check if num ends with 7 or divisible by 7 if(num % 10 == 7 || num % 7 == 0) return true; else return false; } public static void main(String args[]) { int n; Scanner scan = new Scanner(System.in); System.out.print("Enter a number: "); n = scan.nextInt(); //if method returns true then the entered number is //a Buzz number else it is not a Buzz Number if (checkBuzzNumber(n)) System.out.println(n+" is a Buzz number."); else System.out.println(n+" is not a Buzz number."); } }
Output 1: 107 is a Buzz number because it ends with 7.
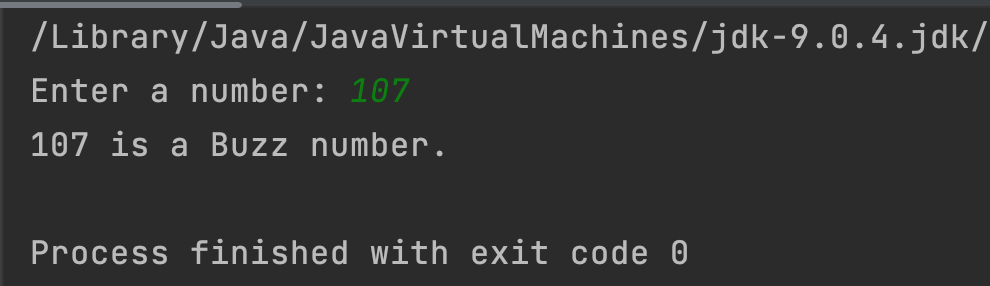
Output 2: 49 is a Buzz number because it is divisible by 7.
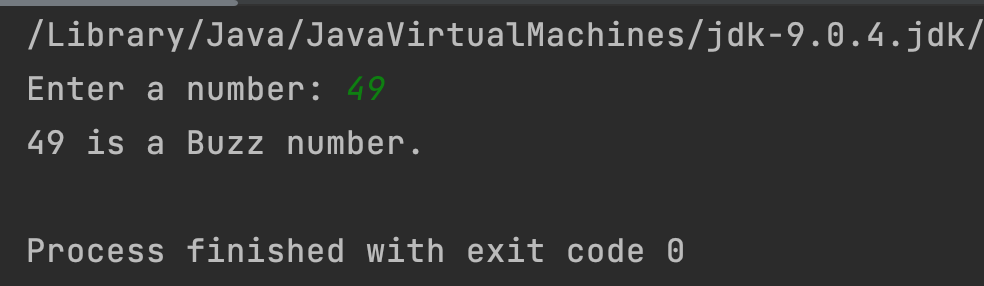
Output 3: 15 is not a Buzz number as it is not divisible by 7 and doesn’t end with digit 7.
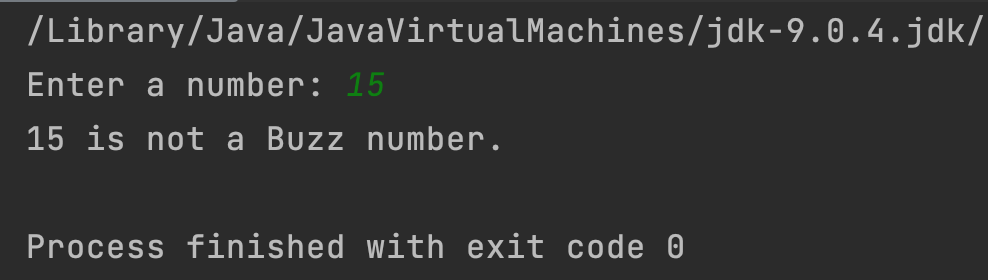
Program to print all Buzz Numbers in a given Range
We are using Scanner to get the lower range and upper range input from the user. The program prints all the numbers between these entered range.
Similar to the previous program, we have a method checkBuzzNumber()
that checks and prints if the number is a Buzz number, we are calling this method inside a for loop to check all the numbers that lies between the given range.
import java.util.Scanner; class JavaExample { public static void main(String args[]) { int lowerRange, upperRange; //Get range input from user Scanner scan = new Scanner(System.in); System.out.print("Enter the lower range: "); lowerRange = scan.nextInt(); System.out.print("Enter the upper range: "); upperRange = scan.nextInt(); System.out.print("Buzz numbers from "+lowerRange+" to "+upperRange+": "); for(int i = lowerRange; i <= upperRange; i++) checkBuzzNumber(i); } // This method will be called for each number between //the entered range, it will print all the Buzz numbers //in the given range static void checkBuzzNumber(int num) { // check if number ends with 7 or divisible by 7 if(num % 10 == 7 || num % 7 == 0) System.out.print(num+ " "); } }
Output 1:

Output 2:
