A positive number that contains a zero digit is called Duck Number. The important point to note is that numbers with only leading zeroes are not Duck numbers. For example, 3056, 10045, 7770 are Duck Numbers while the numbers such as 012, 0045, 01444 are not Duck numbers.
Note: 04505 is also a Duck number as it contains a non-leading zero digit.
Program to Check Duck Number
Here, we have declared a method checkDuckNumber()
, this method checks if the number passed as an argument is Duck number or not.
Inside this method, we are iterating the digits of the given number and checking every digit starting from last digit. If any digit is equal to 0, then it returns true.
import java.util.Scanner; public class JavaExample { // Returns true if the num is a Duck number else it //returns false public static boolean checkDuckNumber(int num) { //check every digit of num starting from the last //digit, at every step remove the last digit after checking while(num != 0) { if(num%10 == 0) return true; num = num / 10; } //if the whole number is processed but the method //didn't return true then it is not a Duck number return false; } public static void main(String args[]) { int n; Scanner scan = new Scanner(System.in); System.out.print("Enter a positive number: "); n = scan.nextInt(); if (checkDuckNumber(n)) System.out.println(n+" is a Duck number."); else System.out.println(n+" is not a Duck number."); } }
Output 1:

Output 2:
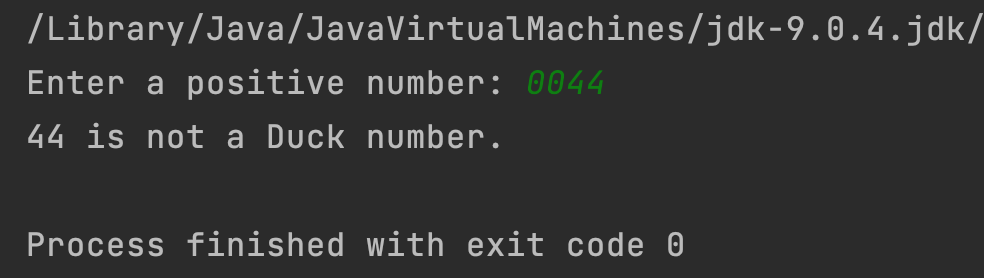
Output 3:
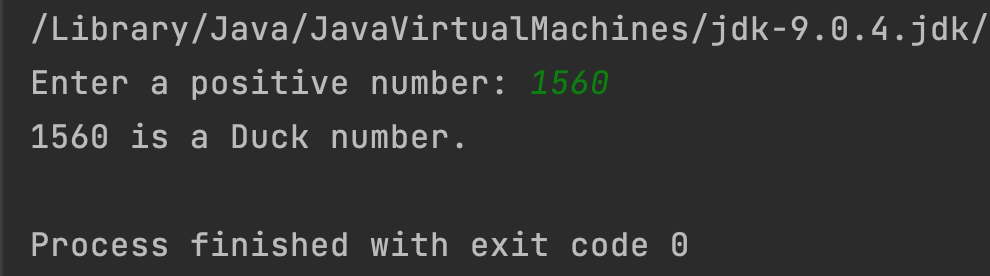
Program to print all Duck numbers in a given range
This program is similar to the above program. Here, user enters the lower range and upper range. The program checks every number between this range and prints all the numbers that are Duck numbers.
import java.util.Scanner; class JavaExample { // Returns true if the number num is a Duck number //else it returns false public static boolean checkDuckNumber(int num) { while(num != 0) { if(num%10 == 0) return true; num = num / 10; } return false; } public static void main(String args[]) { int lowerRange, upperRange; Scanner scan = new Scanner(System.in); System.out.print("Enter the lower range: "); lowerRange = scan.nextInt(); System.out.print("Enter the upper range: "); upperRange = scan.nextInt(); for(int i = lowerRange; i <= upperRange; i++) if(checkDuckNumber(i)){ System.out.print(i+" "); } } }
Output 1:
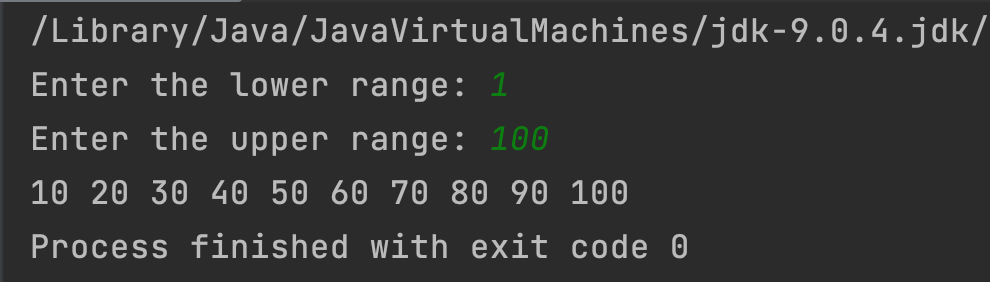
Output 2:
