Before going through exception handling in JSP, let’s understand what is exception and how it is different from errors.
Exception: These are nothing but the abnormal conditions which interrupts the normal flow of execution. Mostly they occur because of the wrong data entered by user. It is must to handle exceptions in order to give meaningful message to the user so that user would be able to understand the issue and take appropriate action.
Error: It can be a issue with the code or a system related issue. We should not handle errors as they are meant to be fixed.
Methods of handling exceptions:
We can handle exceptions using the below two methods.
- Exception handling using exception implicit object
- Exception handling using try catch blocks within scriptlets
Exception handling using exception implicit object
In the below example – we have specified the exception handling page using errorPage attribute of Page directive. If any exception occurs in the main JSP page the control will be transferred to the page mentioned in errorPage attribute.
The handler page should have isErrorPage set to true in order to use exception implicit object. That’s the reason we have set the isErrorPage true for errorpage.jsp.
index.jsp
<%@ page errorPage="errorpage.jsp" %> <html> <head> <title>JSP exception handling example</title> </head> <body> <% //Declared and initialized two integers int num1 = 122; int num2 = 0; //It should throw Arithmetic Exception int div = num1/num2; %> </body> </html>
errorpage.jsp
<%@ page isErrorPage="true" %> <html> <head> <title>Display the Exception Message here</title> </head> <body> <h2>errorpage.jsp</h2> <i>An exception has occurred in the index.jsp Page. Please fix the errors. Below is the error message:</i> <b><%= exception %></b> </body> </html>
output:
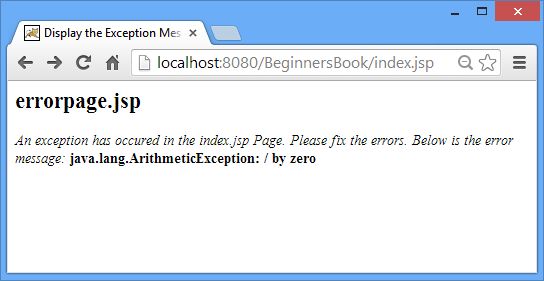
Exception handling using try catch blocks within scriptlets
We have handled the exception using try catch blocks in the below example. Since try catch blocks are java code so it must be placed inside sciptlet. In the below example I have declared an array of length 5 and tried to access the 7th element which doesn’t exist. It caused Array Index out of bounds exception.
error.jsp
<html> <head> <title>Exception handling using try catch blocks</title> </head> <body> <% try{ //I have defined an array of length 5 int arr[]={1,2,3,4,5}; //I'm assinging 7th element to int num //which doesn't exist int num=arr[6]; out.println("7th element of arr"+num); } catch (Exception exp){ out.println("There is something wrong: " + exp); } %> </body> </html>
output of example 2:
Let us know which method do you prefer for handling exceptions and why. If you have any questions, feel free to drop it in the below comment section.
bhaskar says
please add frameworks sir,and add screen shots of outputs.thanks for very stuff of webapplications
sharu says
I cant understand actual difference of jsp and servlet ? we use these technologies seperately or in combine?
shravani says
i prefer to use the exception implicit object method instead of try catch block in scriptlet. because scriptlet is not recommended from long ago as per your previous topics explanation.