Java String valueOf() method is used to convert different types of values to an equivalent String representation. This method is a static method and there are overloaded versions of this method available to handle char
, char[]
, int
, long
, float
, double
, boolean
, Object
, and so on.
Different variants of java string valueOf() method
1. String.valueOf(boolean b)
It takes boolean
value as an argument and returns a String
representation.
boolean flag = true;
String strFlag = String.valueOf(flag);
System.out.println(strFlag); // Output: "true"
2. String.valueOf(char c)
It is used to convert a single char
value to a String
.
char ch = 'Z';
String strChar = String.valueOf(ch);
System.out.println(strChar); // Output: "Z"
3. String.valueOf(char[] data)
It converts a char
array into a String
representation.
char[] chars = {'B', 'o', 'o', 'k'};
String strChars = String.valueOf(chars);
System.out.println(strChars); // Output: "Book"
4. String.valueOf(char[] data, int offset, int count)
Similar to the above method, but it converts a specific portion of char
array to String
. The portion is specified using offset
and count
. Here offset
refers to the from index and count refers to the length. In the following example, the portion of char array is taken from index 1 (offset) till total 2 (count) chars.
char[] chars = {'B', 'o', 'o', 'k'};
String strPartialChars = String.valueOf(chars, 1, 2);
System.out.println(strPartialChars); // Output: "oo"
5. String.valueOf(double d)
It takes double
value as an argument and returns a String
representation.
double d = 6.14149;
String strDouble = String.valueOf(d);
System.out.println(strDouble); // Output: "6.14149"
6. String.valueOf(float f)
It takes float
value as an argument and returns a String
representation.
float f = 3.918f;
String strFloat = String.valueOf(f);
System.out.println(strFloat); // Output: "3.918"
7. String.valueOf(int i)
It takes int
value as an argument and returns a String
representation.
int i = 12;
String strInt = String.valueOf(i);
System.out.println(strInt); // Output: "12"
8. String.valueOf(long l)
It takes long
value as an argument and returns a String
representation.
long l = 123456789L;
String strLong = String.valueOf(l);
System.out.println(strLong); // Output: "123456789"
9. String.valueOf(Object obj)
Converts an Object
to a String
. If the Object
is null
, it returns the string “null”. Otherwise, it returns the result of obj.toString()
.
Object nullObj = null;
String strNullObject = String.valueOf(nullObj);
System.out.println(strNullObject); // Output: "null"
Example 1
Let’s see an example that demonstrates the various versions of String.valueOf()
method.
public class StringValueOfExample {
public static void main(String[] args) {
// boolean to String
boolean flag = true;
String strFlag = String.valueOf(flag);
System.out.println("Boolean to String: " + strFlag);
// char to String
char ch = 'Z';
String strChar = String.valueOf(ch);
System.out.println("Char to String: " + strChar);
// char array to String
char[] chars = {'B', 'o', 'o', 'k'};
String strChars = String.valueOf(chars);
System.out.println("Char array to String: " + strChars);
// specific portion of char array to String
String strPartialChars = String.valueOf(chars, 1, 2);
System.out.println("Partial Char array to String: " + strPartialChars);
// converting double to String
double d = 6.14149;
String strDouble = String.valueOf(d);
System.out.println("Double to String: " + strDouble);
// converting float to String
float f = 3.918f;
String strFloat = String.valueOf(f);
System.out.println("Float to String: " + strFloat);
// converting int to String
int i = 12;
String strInt = String.valueOf(i);
System.out.println("Int to String: " + strInt);
// converting long to String
long l = 123456789L;
String strLong = String.valueOf(l);
System.out.println("Long to String: " + strLong);
// Object to String
Object obj = new Object();
String strObject = String.valueOf(obj);
System.out.println("Object to String: " + strObject);
// null Object to String
Object nullObj = null;
String strNullObject = String.valueOf(nullObj);
System.out.println("Null Object to String: " + strNullObject);
}
}
Example 2
Lets take a simple example to understand the usage of this method. In this example we are concatenating the double nines to the end of the given value.
The given value is an integer, in order to append 99 at the end of the integer we must need to convert the given integer to the string first. We are using valueOf() method to convert the number
to the equivalent string str
and then we are concatenating the 99 at the end of converted string.
public class JavaExample{ public static void main(String args[]){ int number = 23; String str = String.valueOf(number); System.out.println(str+99); } }
Output:
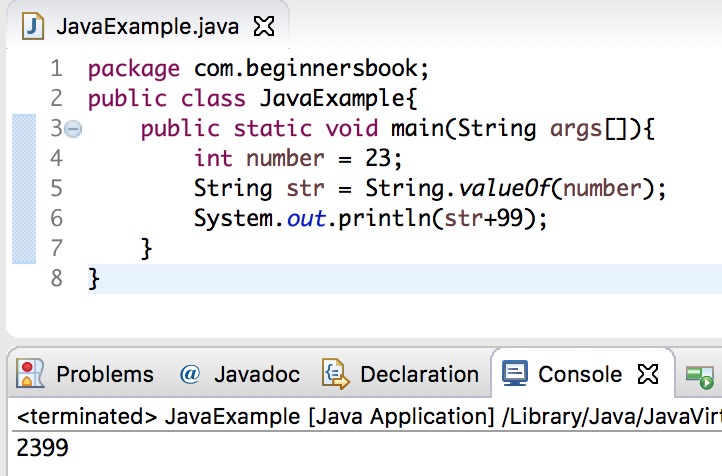
Leave a Reply