The byteValue() method of Integer class returns the given integer value in bytes after narrowing primitive conversion (Integer -> byte) . This method is used when you want to convert the Integer to bytes.
Note: byte range is -128 to 127 and Integer range is -2,147,483,648 to 2,147,483,647 so it is clear that an Integer can hold large values than byte type. This means that this method should be used for smaller integer values else it may given undesired results.
Hierarchy:
java.lang Package -> Integer Class -> byteValue() Method
Syntax of byteValue() method
public byte byteValue()
byteValue() Parameters
- It does not take any parameter.
byteValue() Return Value
- It returns the value contained by Integer object in bytes.
- Return type of byteValue() method is byte.
Supported Java versions: Java 1.5 and onwards
Example 1
class JavaExample { public static void main(String args[]) { // Integer object obj contains value 75 Integer obj = new Integer(75); // converting this Integer value to bytes byte b = obj.byteValue(); // Printing the integer value 75 in bytes System.out.println("Value in bytes: "+b); } }
Output:
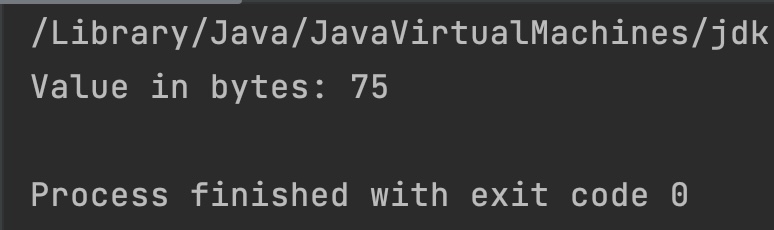
Example 2
If you try to convert an integer value which is out of range (> 127 or < -128) of byte then it may give unexpected negative results. See this: Narrowing primitive conversion.
class JavaExample { public static void main(String args[]) { Integer obj = new Integer(135); byte b = obj.byteValue(); System.out.println("Value of Integer object: "+obj); System.out.println("Value in bytes: "+b); } }
Output:
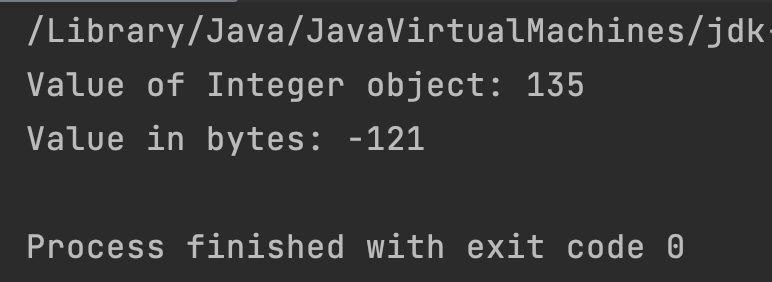
Example 3
In the above examples, we have seen Integer to byte conversion using byteValue(). Let’s see how to convert int to bytes and vice versa.
public class JavaExample{ public static void main(String[] args){ int i = 370; System.out.println("int value: "+i); //int to byte: typecasting is required //since int is > 127, some information is lost byte b = (byte) i; System.out.println("byte value: "+b); //byte to int: No typecasting required i = b; System.out.println("int value: "+i); } }
Output:
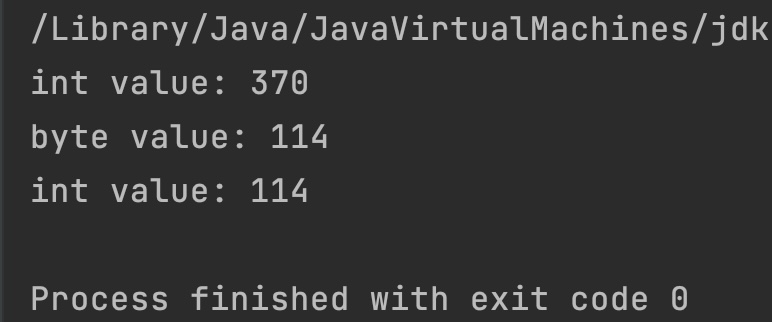