In this tutorial, you will learn how to convert a String to int in Java. If a String is made up of digits like 1,2,3 etc, any arithmetic operation cannot be performed on it until it gets converted into an integer value. In this tutorial we will see the following two ways to convert String to int:
1. Java – Convert String to int using Integer.parseInt(String) method
2. Java – Convert String to int using Integer.valueOf(String) method
1. Java – Convert String to int using Integer.parseInt(String)
The parseInt()
method of Integer wrapper class parses the string as signed integer number. This is how we do the conversion:
Here we have a String str
with the value “1234”, the method parseInt() takes str
as argument and returns the integer value after parsing.
String str = "1234"; int inum = Integer.parseInt(str);
Lets see the complete example:
public class JavaExample{ public static void main(String args[]){ String str="123"; int inum = 100; /* converting the string to an int value * ,the value of inum2 would be 123 after * conversion */ int inum2 = Integer.parseInt(str); int sum = inum+inum2; System.out.println("Result is: "+sum); } }
Output:
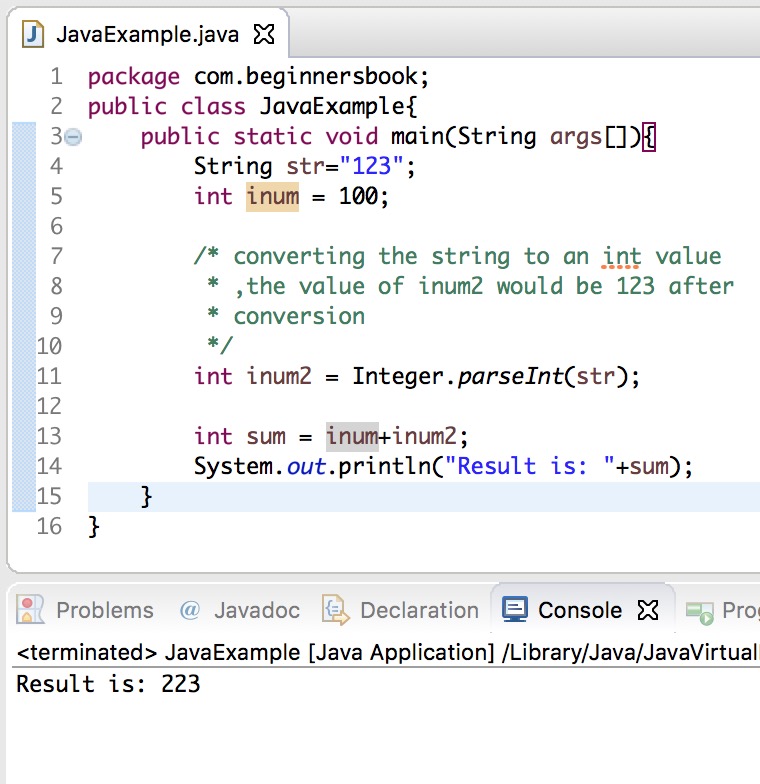
Note: All characters in the String must be digits, however the first character can be a minus ‘-‘ sign. For example:
String str="-1234"; int inum = Integer.parseInt(str);
The value of inum
would be -1234
Integer.parseInt() throws NumberFormatException, if the String is not valid for conversion. For example:
String str="1122ab"; int num = Integer.valueOf(str);
This would throw NumberFormatException
. you would see a compilation error like this:
Exception in thread "main" java.lang.NumberFormatException: For input string: "1122ab" at java.lang.NumberFormatException.forInputString(Unknown Source) at java.lang.Integer.parseInt(Unknown Source) at java.lang.Integer.parseInt(Unknown Source)
Lets see the complete code for String to int conversion.
2. Java – Convert String to int using Integer.valueOf(String)
Integer.valueOf(String)
works same as Integer.parseInt(String)
. It also converts a String to int value. However there is a difference between Integer.valueOf()
and Integer.parseInt()
, the valueOf(String)
method returns an object of Integer class whereas the parseInt(String)
method returns a primitive int value. The output of the conversion would be same whichever method you choose. This is how it can be used:
String str="1122"; int inum = Integer.valueOf(str);
The value of inum would be 1122.
This method also allows first character of String to be a minus ‘-‘ sign.
String str="-1122"; int inum = Integer.valueOf(str);
Value of inum would be -1122.
Similar to the parseInt(String) method it also throws NumberFormatException
when all the characters in the String are not digits. For example a String with value “11aa22” would throw an exception.
Lets see the complete code for conversion using this method.
public class JavaExample{ public static void main(String args[]){ //String with negative sign String str="-234"; //An int variable int inum = 110; /* Convert String to int in Java using valueOf() method * the value of variable inum2 would be negative after * conversion */ int inum2 = Integer.valueOf(str); //Adding up inum and inum2 int sum = inum+inum2; //displaying sum System.out.println("Result is: "+sum); } }
Output:
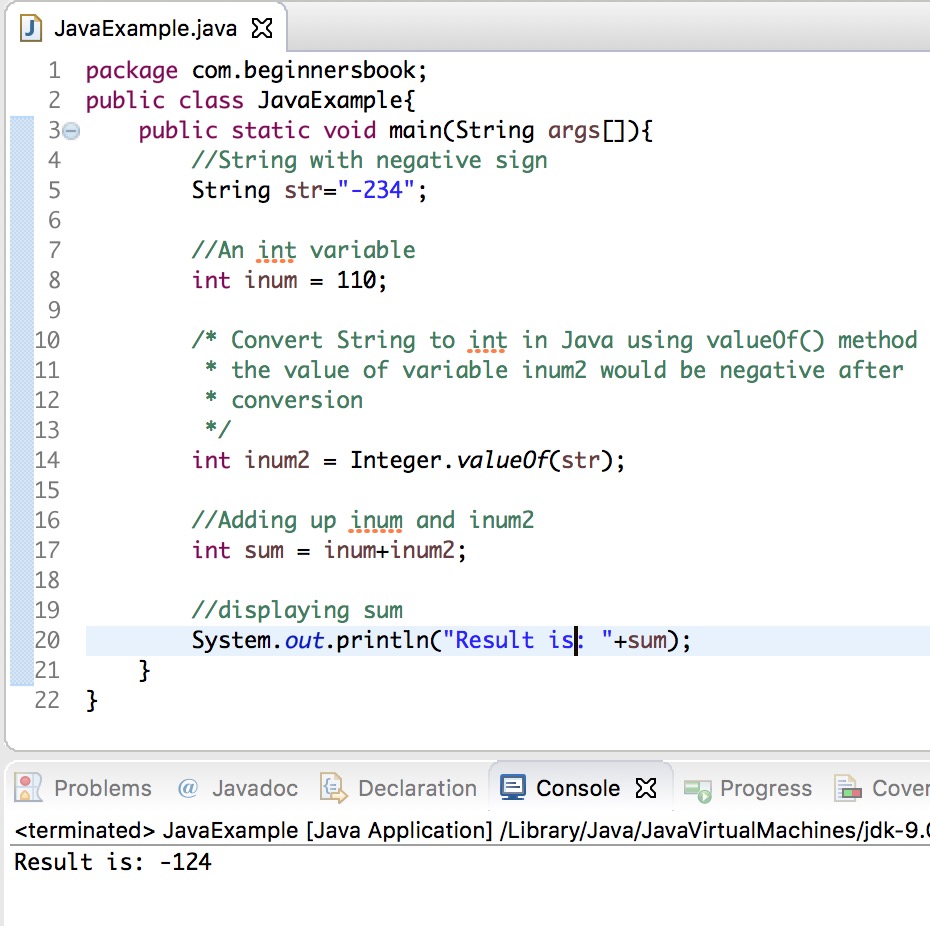
Let’s see another interesting example of String to int conversion.
Convert a String to int with leading zeroes
In this example, we have a string made up of digits with leading zeroes, we want to perform an arithmetic operation on that string retaining the leading zeroes. To do this we are converting the string to int and performing the arithmetic operation, later we are converting the output value to string using format() method.
public class JavaExample{ public static void main(String args[]){ String str="00000678"; /* String to int conversion with leading zeroes * the %08 format specifier is used to have 8 digits in * the number, this ensures the leading zeroes */ str = String.format("%08d", Integer.parseInt(str)+102); System.out.println("Output String: "+str); } }
Output:
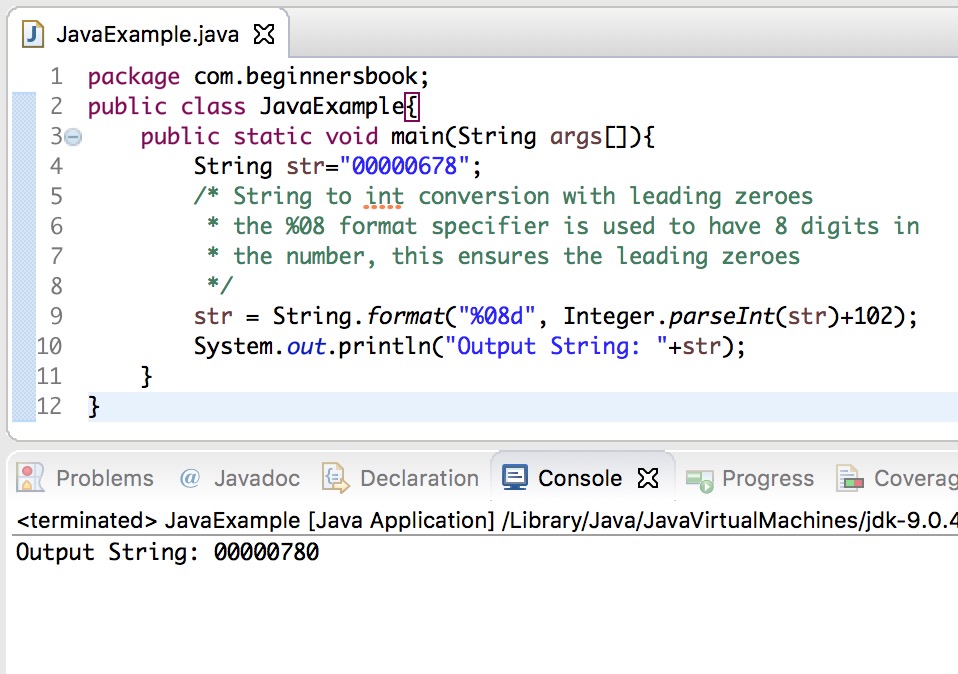
Recommended Posts
- Java int to String Conversion
- Java String to long Conversion
- Java String to double Conversion
- Java String to char Conversion
- Java String to Object Conversion
- Java String to float Conversion
- Java String to Date Conversion
- Java String to boolean Conversion
Leave a Reply