In this tutorial, you will learn how to write java programs to check if a number is Automorphic number or not. A number is called Automorphic, if the square of the number ends with the number itself. For example 25 is an automorphic number because (25)2 = 625. You can see that the square value of 25 ends with the same number 25 (i.e. 625).
In the following image, you can see that the numbers 6 and 25 are Automorphic numbers.
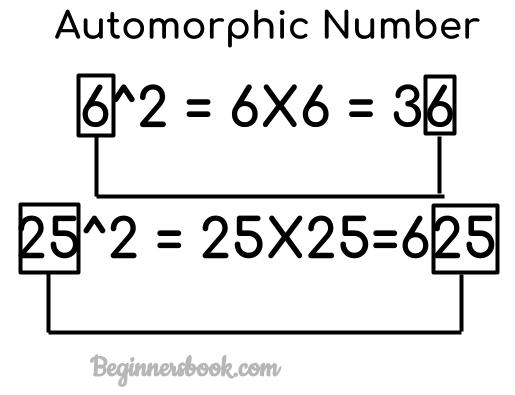
Program to check for Automorphic number
Here we are writing a java program to check if a given number is automorphic. To do this, we have created a user-defined method checkAutomorphic()
, this method takes the number as an input and then returns true if number is an automorphic number else it returns false.
The steps followed in checkAutomorphic() are:
- Find the square of input number.
- Compare the last digit of square with the last digit of number:
- If the last digits are not equal then return false
- If the last digits are equal, proceed to next step.
- Delete the last digit from square and from the number
- Repeat the steps 2 to 4 until the input number becomes zero.
public class JavaExample { //method to check if a number is automorphic static boolean checkAutomorphic(int num) { int square = num * num; while (num > 0) { if (num % 10 != square % 10) return false; num = num/10; square = square/10; } return true; } public static void main(String args[]) { System.out.println("Is 25 automorphic? : "+checkAutomorphic(25)); System.out.println("Is 7 automorphic? : "+checkAutomorphic(7)); } }
Output:
Is 25 automorphic? : true Is 7 automorphic? : false
Java Program to find Automorphic numbers between a given range
In this example, we are displaying all automorphic numbers between a given range. For this, we have created a method to check if the number is equal, similar to the method in above example. However here we have used a different logic, you can use the same logic that we have used above for this.
Then we are running a loop from starting range to ending range and passing each number between this range to the method, we created for checking automorphic number. If method returns true, display that number else skip that number.
Steps are:
- Read starting range and ending range from user.
- Run a loop from starting range till end range and pass each number to the checkAutomorphic() method
- If method returns true display the number else skip it.
import java.util.Scanner; public class JavaExample { private static boolean checkAutomorphic(int num) { int length=0; int square = num*num; //copying the number to a temporary variable int temp = num; //Finding the length of the number while(temp>0) { length++; temp=temp/10; } /* Here we are finding the last few digits of the square * equal to the length of the number. For example, if the number * has two digits then we are finding last two digits of square * if the number has four digits, we are finding last four digits of * square. */ int lastDigits = (int) (square%(Math.pow(10, length))); /* comparing the last digits with the number if equal return * true else returns false */ if (num == lastDigits) return true; else return false; } public static void main(String args[]) { Scanner in = new Scanner(System.in); int first, last; System.out.print("Enter the starting range: "); first = in.nextInt(); System.out.print("Enter the ending range: "); last = in.nextInt(); System.out.println("Automorphic numbers between "+first+" and "+last+" are: "); for(int i=first; i<=last; i++) { if(checkAutomorphic(i)) System.out.print(i+" "); } } }
Output:
Enter the starting range: 5 Enter the ending range: 100 Automorphic numbers between 5 and 100 are: 5 6 25 76
Leave a Reply