Java Math.addExact() method returns sum of its arguments.
public class JavaExample { public static void main(String[] args) { int i = 10, i2 = 20; long l = 10000L, l2 = 15000L; System.out.println(Math.addExact(i, i2)); System.out.println(Math.addExact(l, l2)); } }
Output:
30 25000
Syntax of Math.addExact() method
Math.addExact(5, 7); //returns 12
addExact() Description
public static int addExact(int x, int y): Returns sum of its two integer arguments. It throws ArithmeticException
, if one of the argument is Integer.MAX_VALUE.
public static long addExact(long x, long y): Returns sum of its two long arguments. It throws ArithmeticException
, if one of the argument is Long.MAX_VALUE.
addExact() Parameters
It takes two parameters:
- x: First argument
- y: Second argument
addExact() Return Value
- Returns sum of the first and second argument.
Example 1: Sum of two integer arguments
public class JavaExample { public static void main(String[] args) { int x = 10, y = 20; //two int variables System.out.println("Sum: "+Math.addExact(x, y)); } }
Output:
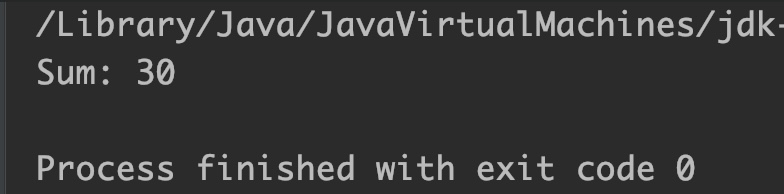
Example 2: Sum of two long arguments
public class JavaExample { public static void main(String[] args) { long x = -10000L, y = 18000L; //two long variables System.out.println("Sum: "+Math.addExact(x, y)); } }
Output:

Example 3: Integer Overflow
public class JavaExample { public static void main(String[] args) { int x = 100, y = Integer.MAX_VALUE; System.out.println(Math.addExact(x, y)); } }
Output:

Example 4: Long Overflow
public class JavaExample { public static void main(String[] args) { long x = 15000L, y = Long.MAX_VALUE; System.out.println(Math.addExact(x, y)); } }
Output:
