Java Math.log(double num) method returns natural logarithm of the double value num
. The natural logarithm is also known as base e logarithm. The e is a constant, whose approximate value is 2.71828.
When base and the value both are equal then log returns 1. Here, we are trying to find out the base e log of value e, result is approximately 1.
public class JavaExample { public static void main(String[] args) { double num = 2.71828; // Base e logarithm of value 2.71828 System.out.println(Math.log(num)); } }
Output:
0.999999327347282
Syntax of Math.log() method
Math.log(10); //returns 2.302585092994046
log() Description
public static double log(double num): Returns the base e logarithm of argument num
. The returns type of log() method is double.
log() Parameters
- num: The double value whose natural logarithm is to be determined.
log() Return Value
- Returns natural logarithm of double value
num
. - If the value of
num
is NaN (Not a number) or less than zero then it returns NaN. - If
num
is positive infinity then it returns positive infinity. - If argument
num
is either positive zero or negative zero then it returns negative infinity.
Example 1: Natural logarithm of numbers 2 and 10
public class JavaExample { public static void main(String[] args) { double num = 2, num2 = 10; System.out.println("Log of 2: "+Math.log(num)); System.out.println("Log of 10: "+Math.log(num2)); } }
Output:

Example 2: Natural logarithm of negative value or NaN
public class JavaExample { public static void main(String[] args) { double num = -2, num2 = 0.0/0; System.out.println("Log of -ve value: "+Math.log(num)); System.out.println("Log of NaN: "+Math.log(num2)); } }
Output:
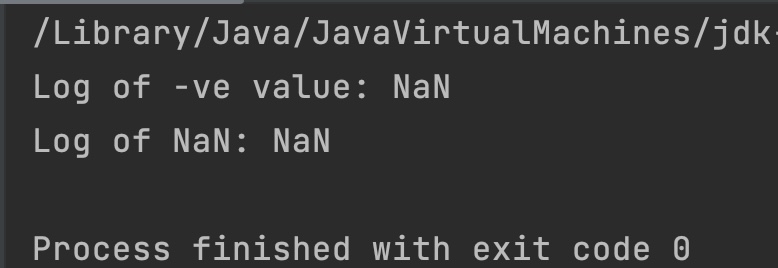
Example 3: Base e logarithm of zero and infinity
public class JavaExample { public static void main(String[] args) { double num = 0, num2 = 10.0/0; System.out.println("Log of zero: "+Math.log(num)); System.out.println("Log of infinity: "+Math.log(num2)); } }
Output:
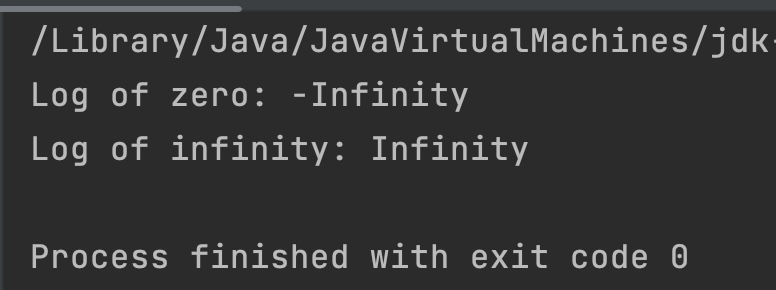