Java Math.incrementExact() method returns the argument after increasing it by one. In this tutorial, we will discuss incrementExact() method with examples.
public class JavaExample { public static void main(String[] args) { int i = 151; //int long l = 8004; //long System.out.println(Math.incrementExact(i)); System.out.println(Math.incrementExact(l)); } }
Output:
152 8005
Syntax of incrementExact() method
Math.incrementExact(1005); //returns 1006
incrementExact() Description
public static int incrementExact(int a): Returns the int argument a after increasing it by one. It throws ArithmeticException, if the result overflows an int (which means if the argument is Integer.MAX_VALUE the increment operation would throw the exception).
public static long incrementExact(long a): Returns the long argument a after increasing it by one. It throws ArithmeticException, if the result overflows a long. This happens when the passed argument is Long.MAX_VALUE.
incrementExact() Parameters
- a: The argument which is an int or long value that needs to be incremented.
incrementExact() Return Value
- Returns a+1 (argument value +1).
Example 1: Increment int value by one
public class JavaExample { public static void main(String[] args) { int i = 151; //int System.out.print("Value of i, incremented by one: "); System.out.println(Math.incrementExact(i)); } }
Output:
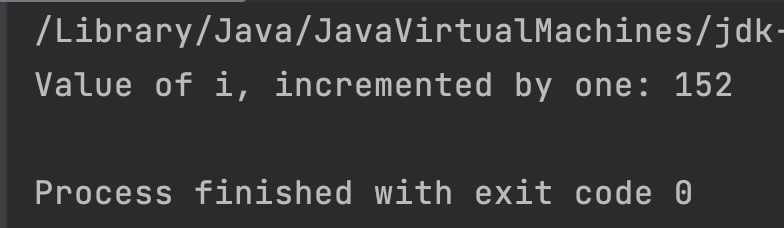
Example 2: Increment long value by one
public class JavaExample { public static void main(String[] args) { long l = 5001; //long System.out.print("Value of l, incremented by one: "); System.out.println(Math.incrementExact(l)); } }
Output:
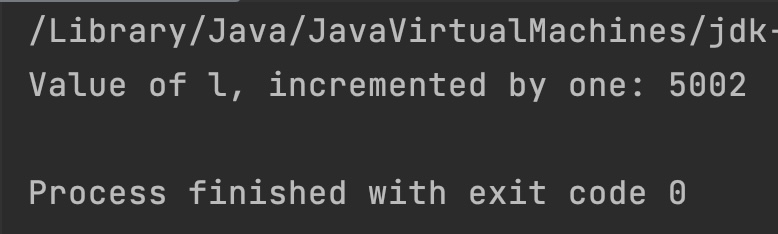
Example 3: Integer overflow while calling incrementExact()
public class JavaExample { public static void main(String[] args) { int l = Integer.MAX_VALUE; System.out.println(Math.incrementExact(l)); } }
Output:

Example 4: Long overflow while calling incrementExact()
public class JavaExample { public static void main(String[] args) { long l = Long.MAX_VALUE; System.out.println(Math.incrementExact(l)); } }
Output:
