Java Math.negateExact() method returns negation of the argument. If the given argument is positive, it returns the same argument with negative sign and vice versa.
public class JavaExample { public static void main(String[] args) { int i = 151; //integer long l = -140056L; //long System.out.println(Math.negateExact(i)); System.out.println(Math.negateExact(l)); } }
Output:
-151 140056
Syntax of negateExact() Method
Math.negateExact(-56); //returns 56
negateExact() Description
public static int negateExact(int num): It returns the negation of integer argument num
. It throws ArithmeticException
, if the argument value is Integer.MIN_VALUE.
public static long negateExact(long num): It returns the negation of long argument num
. It throws ArithmeticException
, if the argument value is Long.MIN_VALUE.
Note: The exception occurs because negation of Integer.MIN_VALUE or Long.MIN_VALUE overflows the int and long respectively.
negateExact() Parameters
- num: The int or long argument whose negation value is to be determined.
negateExact() Return Value
- Returns the negation of given argument
num
.
Example 1: Negation of integer value
public class JavaExample { public static void main(String[] args) { int num = 77; System.out.println(Math.negateExact(num)); } }
Output:
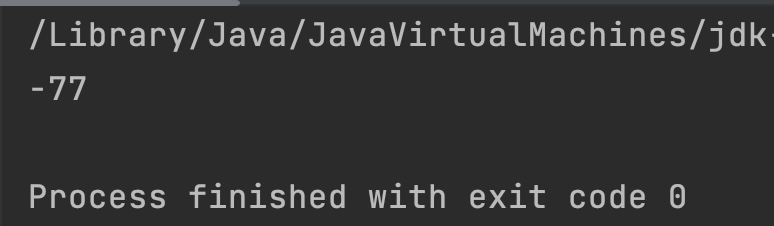
Example 2: Negation of long value
public class JavaExample { public static void main(String[] args) { long num = 4500678L; System.out.println(Math.negateExact(num)); } }
Output:

Example 3: Integer overflow while calling negateExact()
public class JavaExample { public static void main(String[] args) { int num = Integer.MIN_VALUE; System.out.println(Math.negateExact(num)); } }
Output:

Example 4: Long overflow while calling negateExact()
public class JavaExample { public static void main(String[] args) { long num = Long.MIN_VALUE; System.out.println(Math.negateExact(num)); } }
Output:
