Java Math.toIntExact() method returns the long argument as an int. This method accepts long data type value as an argument and returns the equivalent integer value.
public class JavaExample { public static void main(String[] args) { long num = -1600568L; //converts long to int int i = Math.toIntExact(num); System.out.println(i); } }
Output:
-1600568
Syntax of Math.toIntExact() method
Math.toIntExact(1001L); //returns 1001
toIntExact() method Description
public static int toIntExact(long num): Returns the long argument as an int. It throws ArithmeticException, if the result overflows an int.
toIntExact() Parameters
- num: The long value passed as an argument.
toIntExact() Return Value
- The num value as an int.
Example 1: Long to int conversion using toIntExact()
public class JavaExample { public static void main(String[] args) { long num = 500444L; int i = Math.toIntExact(num); System.out.println(Math.toIntExact(i)); } }
Output:
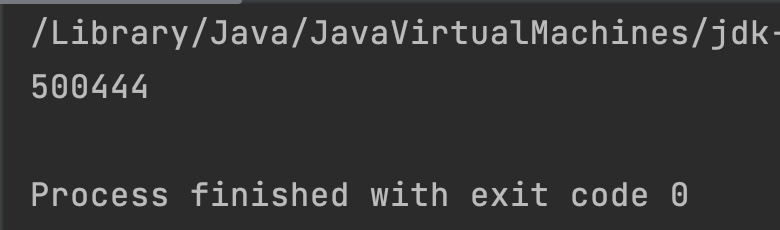
Example 2: Integer overflow while calling toIntExact()
public class JavaExample { public static void main(String[] args) { long num = Long.MAX_VALUE; int i = Math.toIntExact(num); System.out.println(Math.toIntExact(i)); } }
Output:

Example 3: If Long.MIN_VALUE passed as an argument
public class JavaExample { public static void main(String[] args) { long num = Long.MIN_VALUE; int i = Math.toIntExact(num); System.out.println(Math.toIntExact(i)); } }
Output:
