In this tutorial, we will write a java program to print the Left Triangle Star Pattern. This is also called mirrored Right Triangle Star Pattern.
This is how a left triangle pattern looks like:
Number of rows = 6 Output: * * * * * * * * * * * * * * * * * * * * *
Program to print Left Triangle Star Pattern
In this program we are displaying the left triangle star pattern. We are working with three main variables here, row
represents the rows of the pattern, column
represents the columns of the pattern and numberOfRows
that represents the number of the rows that needs to be printed.
Logic used in the program to print Left Triangle Star pattern:
Here we have main for loop which runs from 0 till numberOfRows
and inside this main loop, we have two for loops.
The first loop is to print the spaces and the second loop is to print the stars (*) in the output. You may be wondering why we are using 2 multiplier (i.e. 2*(numberOfRows-row)) in the for loop. This is because we are already leaving a space between stars, so for the first row we need to give 10 spaces (5 for stars and 5 for in between star spaces).
The second loop is pretty self explanatory as this prints one star in first row, two stars in second row and so on.
public class JavaExample { public static void main(String args[]) { int row, column, numberOfRows = 6; for (row=0; row<numberOfRows; row++) { for (column=2*(numberOfRows-row); column>2; column--) { System.out.print(" "); } for (column=0; column<=row; column++ ) { System.out.print("* "); } //To move cursor to new line for each row System.out.println(); } } }
Output:
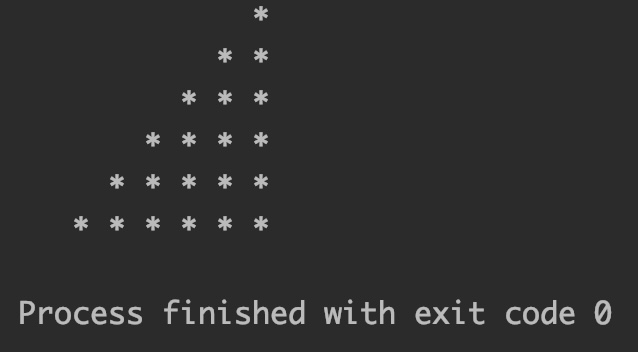
Leave a Reply