In this article, you will learn how to write a Java Program to print left Pascal triangle pattern.
Left Pascal Triangle Pattern Illustration:
Number of rows = 6 Output: * ** *** **** ***** ****** ***** **** *** ** *
Example 1: Program to print Left Pascal Triangle Star Pattern
public class JavaExample { public static void main(String[] args) { //Initializing number of rows in the pattern //This represents the row with the max stars int numberOfRows= 6; //There are two outer for loops in this program //This is Outer Loop 1: This prints the first half of // the Left Pascal triangle pattern for (int i= 1; i<=numberOfRows; i++) { //Prints the whitespaces before the first star of each row for (int j=i; j<numberOfRows; j++) { System.out.print(" "); } //Prints the stars of each row for (int k=1; k<=i;k++) { System.out.print("*"); } //To move the cursor to new line for next row System.out.println(); } //Outer Loop 2: Prints second half of the triangle for (int i=numberOfRows; i>=1; i--) { //Prints the whitespaces before the first star of each row for(int j=i; j <=numberOfRows;j++) { System.out.print(" "); } //Prints stars of each row for(int k=1; k<i ;k++) { System.out.print("*"); } //Move the cursor to new line after printing a row System.out.println(); } } }
Output:
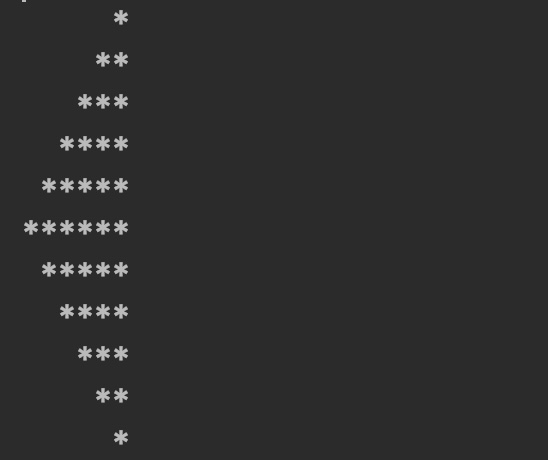
Example 2: Print Left Pascal Triangle Pattern based on user input
The following program is same as the above program except that here the number of rows is entered by user, which is captured in this program using Scanner.
import java.util.Scanner; public class JavaExample { public static void main(String[] args) { //This represents the row with the max stars int numberOfRows; System.out.println("Enter the number of rows: "); //Getting the number of rows from user Scanner sc = new Scanner(System.in); numberOfRows = sc.nextInt(); //This is Outer Loop 1: This prints the first half of // the pattern for (int i= 1; i<=numberOfRows; i++) { //Prints the spaces before the first star of each row for (int j=i; j<numberOfRows; j++) { System.out.print(" "); } //Prints the stars for each row for (int k=1; k<=i;k++) { System.out.print("*"); } //To move the cursor to new line after printing row System.out.println(); } //Outer Loop 2: Prints second half of the triangle for (int i=numberOfRows; i>=1; i--) { //Prints the whitespaces before the first star of each row for(int j=i; j <=numberOfRows;j++) { System.out.print(" "); } //Prints stars of each row for(int k=1; k<i ;k++) { System.out.print("*"); } //To move the cursor to new line after printing row System.out.println(); } } }
Output:
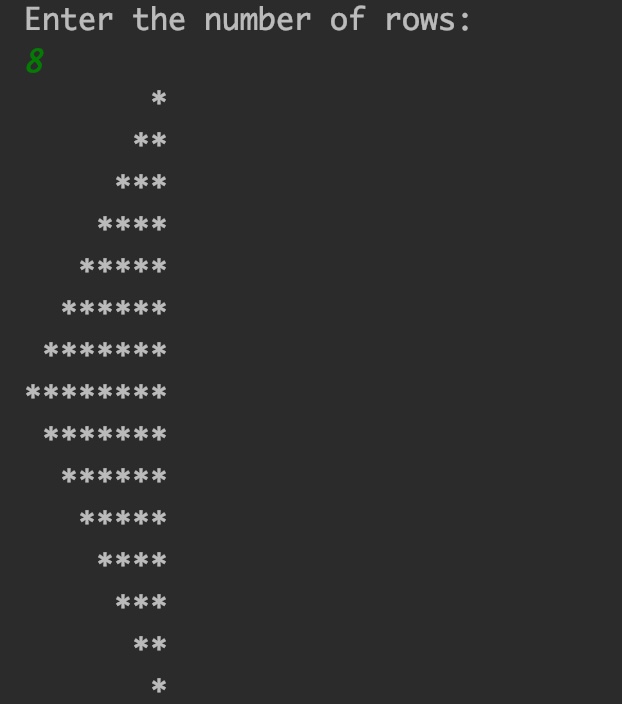
Leave a Reply