In this article, you will learn how to write a java program to print reverse Pyramid Star Pattern.
This is how a reverse pyramid pattern using stars looks:
Number of rows = 7
Output:
* * * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
Example 1: Program to Print Reverse Pyramid Pattern
public class JavaExample { public static void main(String[] args) { int numberOfRows=7; //This loop runs based on the number of rows //In this case, the loop runs 7 times to print 7 rows for (int i= 0; i<= numberOfRows-1; i++) { //This loop prints starting spaces for each row of pattern for (int j=0; j<=i; j++) { System.out.print(" "); } //This loop prints stars and the space between stars for each row for (int k=0; k<=numberOfRows-1-i; k++) { System.out.print("*" + " "); } //To move the cursor to new line after each row System.out.println(); } } }
Output:
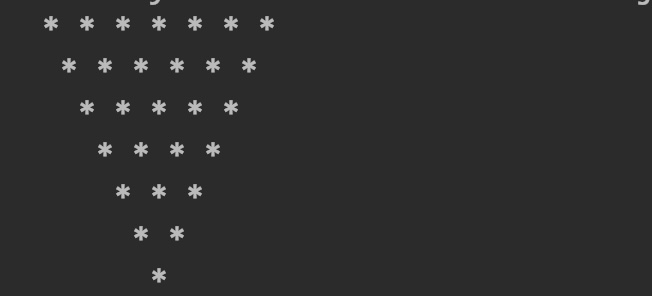
Example 2: Program to Print Reverse Pyramid Pattern based on user input
In the following program, the number of rows is entered by the user and the program gets that value using Scanner. The value then used by for loop to print the pattern consisting user entered number of rows.
import java.util.Scanner; public class JavaExample { public static void main(String[] args) { int numberOfRows; System.out.println("Enter Number of rows: "); //Getting the number of rows input from user Scanner sc = new Scanner(System.in); numberOfRows = sc.nextInt(); sc.close(); //This loop will run based on the input by user //if user enters 5 then the loop will run 5 times //if user enters 8 then the loop will run 8 times for (int i= 0; i<= numberOfRows-1; i++) { //Print spaces before first star of each row for (int j=0; j<=i; j++) { System.out.print(" "); } //Print stars and spaces between them for (int k=0; k<=numberOfRows-1-i; k++) { System.out.print("*" + " "); } //new line for each row System.out.println(); } } }
Output: User enters number 8 so the program prints 8 rows reverse pyramid pattern.
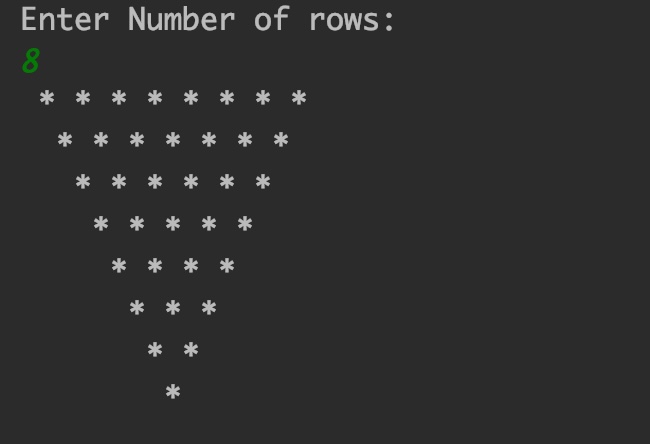
Leave a Reply