Java Math multiplyExact() method returns the product of its arguments. In this tutorial, we will discuss multiplyExact() method with examples.
public class JavaExample { public static void main(String[] args) { int i = 20, i2 = 10; long l = 10000L, l2 = 15000L; System.out.println(Math.multiplyExact(i, i2)); System.out.println(Math.multiplyExact(l, l2)); } }
Output:
200 150000000
Syntax of multiplyExact() method
Math.multiplyExact(10, 20); //returns 200
multiplyExact() Description
public static int multiplyExact(int x, int y): Returns the product of integer arguments x and y. It throws an ArithmeticException if the result overflows an int (which means if result is less than Integer.MIN_VALUE or greater than Integer.MAX_VALUE).
public static long multiplyExact(long x, long y): Returns the product of long arguments x and y. It throws an ArithmeticException if the result overflows a long (which means if result is less than Long.MIN_VALUE or greater than Long.MAX_VALUE).
public static long multiplyExact(long x, int y): This variation of multiplyExact() method is introduced in JDK 9 so it won’t run on older java versions. It returns the product of its arguments. It throws an ArithmeticException if the result overflows a long.
multiplyExact() Parameters
- x: First argument
- y: Second argument
multiplyExact() Return Value
- Returns the product of x and y.
Example 1: Multiplication of two integer arguments
public class JavaExample { public static void main(String[] args) { int x = 100, y = 3; System.out.println(Math.multiplyExact(x, y)); } }
Output:
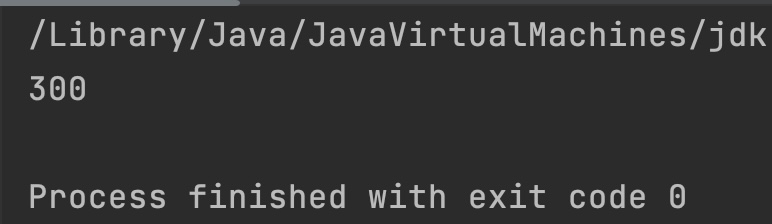
Example 2: Multiplication of two long arguments
public class JavaExample { public static void main(String[] args) { long x = 1000L, y = 3000L; System.out.println(Math.multiplyExact(x, y)); } }
Output:

Example 3: Integer overflow
public class JavaExample { public static void main(String[] args) { int x = 100, y = Integer.MAX_VALUE; System.out.println(Math.multiplyExact(x, y)); } }
Output:

Example 4: Long overflow
public class JavaExample { public static void main(String[] args) { long x = 1000L, y = Long.MIN_VALUE; System.out.println(Math.multiplyExact(x, y)); } }
Output:
