In this tutorial, you will learn how to write a java program to print Sandglass star pattern.
Sandglass star Pattern Illustration:
Number of rows: 5 Output: * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
Example 1: Program to print Sandglass star Pattern
public class JavaExample { public static void main(String[] args) { //Initialized the number of rows, this represents //the number of rows in first half and second half //of the Sandglass pattern int numberOfRows = 6; //Outer loop 1: Prints the first half of the pattern for (int i= 0; i<= numberOfRows-1 ; i++) { //Prints the spaces before the first star of each row for (int j=0; j<i; j++) { System.out.print(" "); } //Prints stars and the whitespaces in between them for (int k=i; k<=numberOfRows-1; k++) { System.out.print("*" + " "); } //Next line for new row System.out.println(); } //Outer loop 2: Prints the second half of the pattern for (int i= numberOfRows-1; i>= 0; i--) { //Prints the spaces before the first star of each row //of the second half of the pattern for (int j=0; j<i; j++) { System.out.print(" "); } //Prints stars and the whitespaces in between them for (int k=i; k<=numberOfRows-1; k++) { System.out.print("*" + " "); } //Move the cursor to new line for next row System.out.println(); } } }
Output:
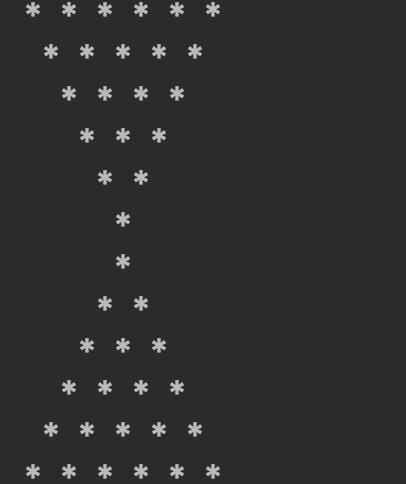
Example 2: Print Sandglass star pattern based on user Input
This program is same as the program shown in first example, except that here the number of rows is not hardcoded and is entered by user during runtime.
import java.util.Scanner; public class JavaExample { public static void main(String[] args) { //This represents the number of rows in first half a // and second half of the Sand glass pattern int numberOfRows; //Getting the number of rows from user System.out.println("Enter the number of rows: "); Scanner sc = new Scanner(System.in); numberOfRows = sc.nextInt(); sc.close(); //Outer loop 1: Prints the first half of the pattern for (int i= 0; i<= numberOfRows-1 ; i++) { //Inner loop 1: Prints the spaces before the first star of each row for (int j=0; j<i; j++) { System.out.print(" "); } //Inner loop 2: Prints stars and the whitespaces in between them for (int k=i; k<=numberOfRows-1; k++) { System.out.print("*" + " "); } //Next line for new row System.out.println(); } //Outer loop 2: Prints the second half of the pattern for (int i= numberOfRows-1; i>= 0; i--) { //Inner loop 1: Prints spaces before first star of the row for (int j=0; j<i; j++) { System.out.print(" "); } //Inner loop 2: Prints stars and the whitespaces in between them for (int k=i; k<=numberOfRows-1; k++) { System.out.print("*" + " "); } //Move the cursor to new line for next row System.out.println(); } } }
Output:
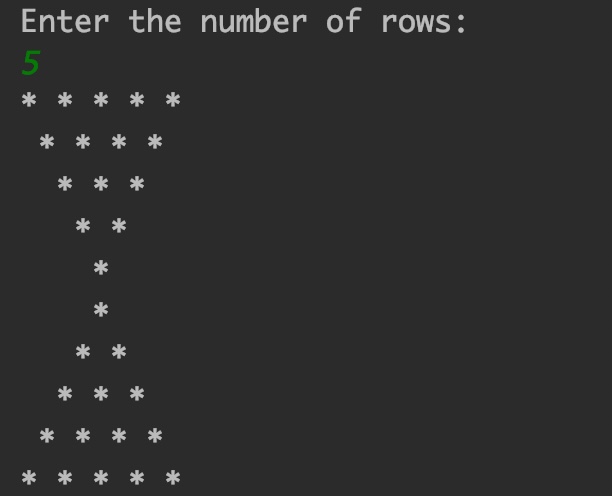
Leave a Reply