In this article, you will learn how to write a Java program to print hollow square star pattern.
Hollow Square star pattern illustration:
Number of rows: 5 Output: * * * * * * * * * * * * * * * *
Example 1: Print hollow square star pattern using for loop
In this example, we are using for loop to print the hollow square pattern based on the user input. User enters the max stars in each row, these stars represents the size of the each side of the square. Here user enters number 5 which means each side of the square consists of 5 stars in the output.
import java.util.Scanner; public class JavaExample { public static void main(String[] args) { //Getting the size of the square side from user Scanner sc = new Scanner(System.in); System.out.print("Enter the stars in each side of square: "); int sideSize = sc.nextInt(); System.out.println("Hollow Square Star Pattern: "); for (int i = 0; i < sideSize; i++ ) { for (int j = 0 ; j < sideSize; j++ ) { if (i == 0 || i == sideSize - 1 || j == 0 || j == sideSize - 1) { System.out.print("*"+" "); } else { // Double spaces are to accommodate space between the stars // For example, when square side size is 5, the total spaces // in 2nd row, between first and last star are: // 7 (3 for stars + 4 for spaces between stars) System.out.print(" "+ " "); } } //To move the cursor to new line System.out.println(); } } }
Output:
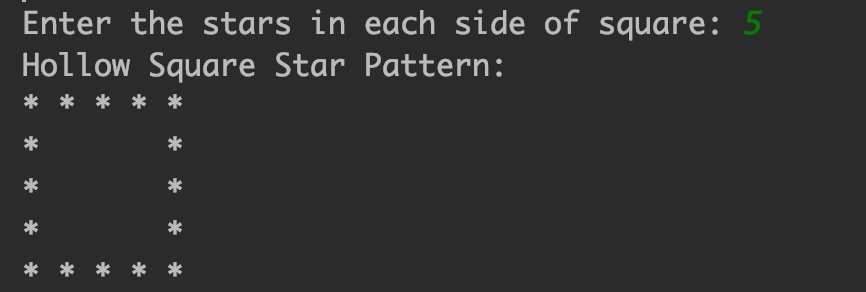
Example 2: Print hollow square star pattern using while loop
In the following example, we are using while loop to print the pattern. This is same as the first example except here we are using the same logic using while loop.
import java.util.Scanner; public class JavaExample { public static void main(String[] args) { int i=0, j; //Getting the size of the square side from user Scanner sc = new Scanner(System.in); System.out.print("Enter the stars in each side of square: "); int sideSize = sc.nextInt(); System.out.println("Hollow Square Star Pattern: "); while (i < sideSize ) { j = 0 ; while ( j < sideSize ) { if (i == 0 || i == sideSize - 1 || j == 0 || j == sideSize - 1) { System.out.print("*"+ " "); } else { System.out.print(" "+ " "); } j++; } //To move cursor to new line for next row System.out.println(); i++; } } }
Output:
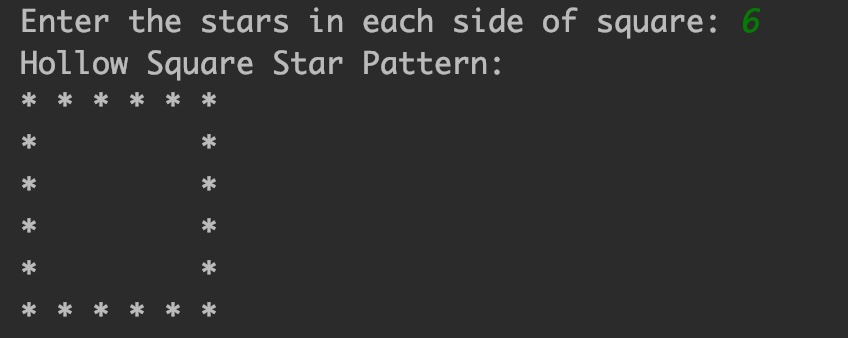
Leave a Reply