In this tutorial, you will learn how to sort ArrayList in Java. We will write several java programs to accomplish this. We can use Collections.sort()
method to sort an ArrayList in ascending and descending order.
//Sorting in Ascending order
ArrayList<Integer> numbers = new ArrayList<>(List.of(4, 1, 3, 2));
Collections.sort(numbers); // Sort the ArrayList in ascending order
System.out.println(numbers); // Output: [1, 2, 3, 4]
//Sorting in Descending order
ArrayList<Integer> numbers = new ArrayList<>(List.of(4, 1, 3, 2));
// Sort the ArrayList in descending order
Collections.sort(numbers, Collections.reverseOrder());
System.out.println(numbers); // Output: [4, 3, 2, 1]
Let’s see the complete programs:
Example 1: Sorting ArrayList in Ascending order
In this example, we have an ArrayList
of String type. We are sorting the given ArrayList in ascending order using Collections.sort()
method. Since this ArrayList is of string type, the elements are sorted in ascending alphabetical order.
import java.util.*; public class JavaExample { public static void main(String args[]){ ArrayList<String> listOfCountries = new ArrayList<>(); listOfCountries.add("India"); listOfCountries.add("US"); listOfCountries.add("China"); listOfCountries.add("Denmark"); // Original unsorted list System.out.println("Before Sorting: "+ listOfCountries); //Sorting the ArrayList using sort() method Collections.sort(listOfCountries); // Printing sorted ArrayList System.out.println("After Sorting: "+ listOfCountries); } }
Output:

Example 2: Sorting ArrayList of Integer Type
Let’s see the same program but with different type of ArrayList. Here, we are sorting an ArrayList of integers.
import java.util.*; public class JavaExample{ public static void main(String args[]){ ArrayList<Integer> arraylist = new ArrayList<>(); arraylist.add(11); arraylist.add(2); arraylist.add(7); arraylist.add(3); //Before sorting System.out.println("Before Sorting: "+ arraylist); // Sorting the list of integers using sort() method Collections.sort(arraylist); //After sorting System.out.println("After Sorting: "+ arraylist); } }
Output:
Before Sorting: [11, 2, 7, 3] After Sorting: [2, 3, 7, 11]
Example 3: Sorting an ArrayList in Descending order
In this program, we are sorting the given ArrayList in descending order. To sort an ArrayList in descending order, we need to pass Collection.reverseOrder()
as a second parameter in the Collections.sort()
method as shown below. The same way, we can sort an ArrayList of integer type in descending order as well.
import java.util.*; public class JavaExample{ public static void main(String args[]){ ArrayList<String> arraylist = new ArrayList<>(); arraylist.add("Apple"); arraylist.add("Orange"); arraylist.add("Kiwi"); arraylist.add("Banana"); arraylist.add("Mango"); //Before sorting System.out.println("Before Sorting: "+ arraylist); // Sorting the list in descending order Collections.sort(arraylist, Collections.reverseOrder()); //After sorting System.out.println("After Sorting: "+ arraylist); } }
Output:

Example 4: Sort ArrayList entered by user in descending order
In this example, user is asked to enter the size of ArrayList. This is read and stored in an int variable size
. A while loop runs to read and add the user entered elements to the ArrayList. Once the list is populated with the user entered elements, the list is sorted in reverse order by using the sort()
method similar to the Example 3.
import java.util.*; public class JavaExample { public static void main(String[] args) { int i=0, size; Scanner scan = new Scanner(System.in); ArrayList<String> list = new ArrayList<>(); System.out.println("How many elements you want in list? "); size = scan.nextInt(); Scanner scan2 = new Scanner(System.in); System.out.println("Enter elements: "); while(i<size) { list.add(scan2.nextLine()); i++; } //Before sorting System.out.println("Before Sorting: "+ list); // Sorting the list in descending order Collections.sort(list, Collections.reverseOrder()); //After sorting System.out.println("After Sorting: "+ list); } }
Output:
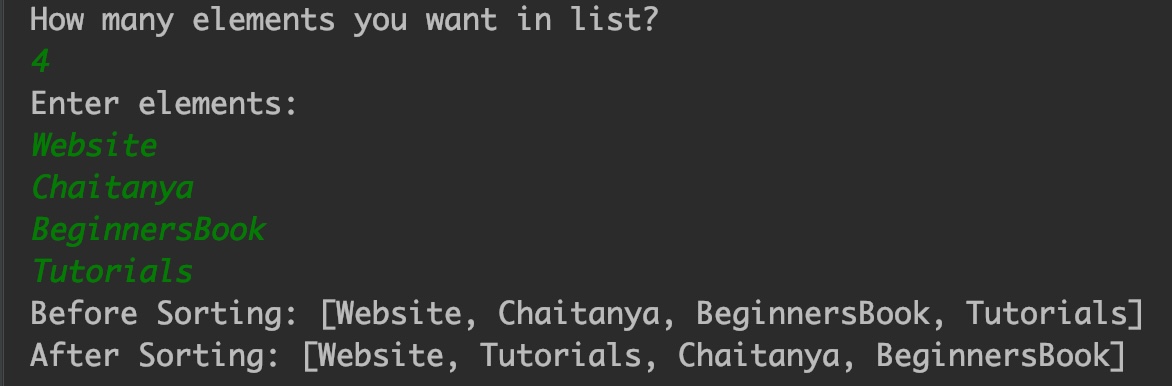
Related guides:
papajo says
Hi, good article, but will Collections.sort eliminate duplicates in case the list contains any duplicates? If not can you show how to eliminate the duplicates?
Chaitanya Singh says
Refer this: https://beginnersbook.com/2014/10/how-to-remove-repeated-elements-from-arraylist/
Frank says
For the main method it is supposed to be (String[] args) not (String args[])
Chaitanya Singh says
Hello Frank, Both are same.
Gopal says
Great tutorial, even for beginners. Sorted my array immediately.
ni says
One question, does .sort retain existing order? This is often relevant. If you have it sorted by one criteria, then sort it by another criteria, do values by the second criteria which are equal still show the initial sort criteria is retained, or is the resulting order random?