There are several ways to convert ArrayList to HashSet. In this guide, we will discuss the following ways with examples:
- Passing ArrayList to constructor of HashSet class
- Iterating ArrayList and adding elements to HashSet using HashSet.add()
- Using HashSet.addAll() method
- Using java 8 stream
1. Passing ArrayList to constructor of HashSet class
One of the constructor of HashSet accepts a collection as an argument. Here, we have passed the ArrayList that we have created to the HashSet constructor. This copies all the elements of ArrayList to HashSet.
import java.util.HashSet; import java.util.ArrayList; class JavaExample { public static void main(String[] args) { ArrayList<String> arrList = new ArrayList<>(); arrList.add("C"); arrList.add("C++"); arrList.add("Java"); arrList.add("Python"); System.out.println("ArrayList Elements: "+arrList); // Passing the arrList to HashSet constructor // This will copy all the elements of ArrayList to HashSet HashSet<String> hSet = new HashSet<>(arrList); System.out.println("HashSet Elements: "); // Displaying HashSet elements for (String s : hSet) { System.out.println(s); } } }
Output:
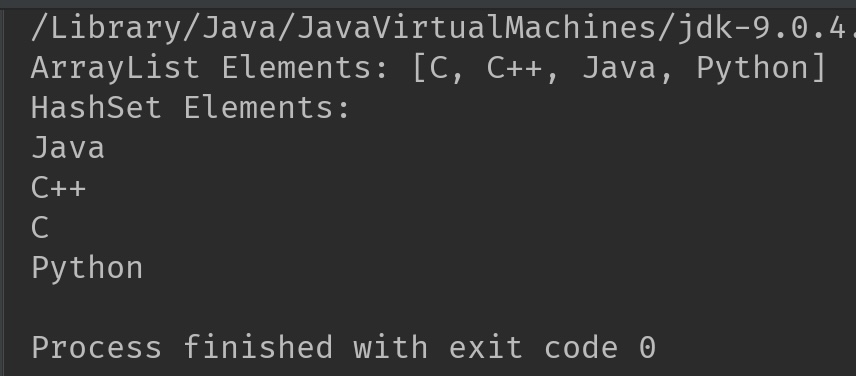
2. Iterating ArrayList and adding elements to HashSet using HashSet.add()
Iterating the ArrayList using forEach() method and adding the element to the corresponding HashSet on every iteration.
import java.util.HashSet; import java.util.ArrayList; class JavaExample { public static void main(String[] args) { ArrayList<String> arrList = new ArrayList<>(); arrList.add("C"); arrList.add("C++"); arrList.add("Java"); arrList.add("Python"); // Creating an empty HashSet HashSet<String> hSet = new HashSet<>(); // Adding each element of ArrayList element to HashSet arrList.forEach(item -> { hSet.add(item); }); System.out.println("HashSet Elements: "); // Displaying HashSet elements for (String s : hSet) { System.out.println(s); } } }
Output:
HashSet Elements: Java C++ C Python
3. Using HashSet.addAll() method
Here, we are using the addAll() method of HashSet class to convert ArrayList to HashSet. In this example, we have an ArrayList of Integer type and we are adding its elements to HashSet of Integer type. In the previous examples, we have seen the conversion of String type ArrayList to HashSet. This time we are converting Integer type ArrayList so notice all the places where we have made changes from String to Integer in the program.
import java.util.HashSet; import java.util.ArrayList; class JavaExample { public static void main(String[] args) { ArrayList<Integer> arrList = new ArrayList<>(); arrList.add(11); arrList.add(22); arrList.add(33); arrList.add(44); // Creating an empty HashSet HashSet<Integer> hSet = new HashSet<>(); // Adding all elements of ArrayList in one go // The addAll() method copies all elements of a collection // to HashSet. hSet.addAll(arrList); System.out.println("HashSet Elements: "); // Displaying HashSet elements for (int num : hSet) { System.out.println(num); } } }
Output:
HashSet Elements: 33 22 11 44
4. Using java 8 stream
Java 8 introduced the concept of Streams. Here, we are using stream to convert ArrayList to HashSet.
import java.util.ArrayList; import java.util.HashSet; import java.util.stream.*; class JavaExample { public static void main(String[] args) { ArrayList<String> arrList = new ArrayList<>(); arrList.add("AA"); arrList.add("BB"); arrList.add("CC"); arrList.add("DD"); // using java 8 stream to convert the ArrayList to // HashSet. Notice the typecast HashSet<String> HashSet<String> hSet = (HashSet<String>)arrList.stream().collect( Collectors.toSet()); System.out.println("HashSet Elements: "); // Displaying HashSet elements for (String s : hSet) { System.out.println(s); } } }
Output:
HashSet Elements: AA BB CC DD