In this guide, we will see how to convert Integer List to int Array in Java. There are several ways, you can do this conversion, we have covered following methods:
- Using toArray() method
- Using simple for loop
- Using Stream API
1. Using toArray() method
This is one of the simplest way of doing the ArrayList to Array conversion. Here, we are using toArray() method of ArrayList class to convert the given Integer ArrayList to int array.
import java.util.*; public class JavaExample { public static void main(String[] args) { //A List of integers List<Integer> list= new ArrayList<>(); list.add(2); list.add(4); list.add(6); list.add(8); list.add(10); System.out.println("List Elements: "+list); // List to Array Conversion using toArray() Object arr[]=list.toArray(); System.out.println("Array Elements: "); //Print array elements for(Object num : arr) { System.out.println(num); } } }
Output:
List Elements: [2, 4, 6, 8, 10] Array Elements: 2 4 6 8 10
2. Using Simple for loop
In this example, we are using a simple for loop to convert Integer list to int array. We are iterating the list using for loop and assigning the list elements to array at every iteration. To fetch the list elements based on index, we are using get() method.
The steps are as follows:
- Create an array of same size. The size of list is obtained using size() method.
- Loop the list and get element at every iteration.
- Assign the element fetched from the list to the int array.
- Print array elements.
import java.util.*; public class JavaExample { public static void main(String[] args) { //ArrayList declaration and initialization List<Integer> arrList= Arrays.asList(2, 4, 8, 16); //creating an array of same size int arr[] = new int[arrList.size()]; //iterating ArrayList and adding each element //manually using get() method. for(int j =0;j<arrList.size();j++){ arr[j] = arrList.get(j); } System.out.println("List Elements: "+ arrList); System.out.println("Array Elements:"); //Displaying Array elements for(int i: arr) { System.out.println(i); } } }
Output:
List Elements: [2, 4, 8, 16] Array Elements: 2 4 8 16
3. Using Steam API
Here, we are using java 8 stream API. The steps followed in this program are:
- Convert the
List<String>
toStream<String>
using thestream()
method. - Convert
Stream<String>
toStream<Integer>
usingmapToInt()
method. - Convert
Stream<Integer>
to int array usingtoArray()
method.
import java.util.*; class JavaExample { public static void main(String[] args) { // List of Integer type List<Integer> arrList = new ArrayList<>(); arrList.add(1); arrList.add(3); arrList.add(5); arrList.add(7); arrList.add(9); System.out.println("ArrayList Elements: "+arrList); // stream() converts given ArrayList to stream // mapToInt() converts the obtained stream to IntStream // toArray() is used to return an array int[] arr = arrList.stream() .mapToInt(Integer::intValue) .toArray(); System.out.println("Array Elements: "); // Print array elements for(int i: arr){ System.out.println(i); } } }
Output:
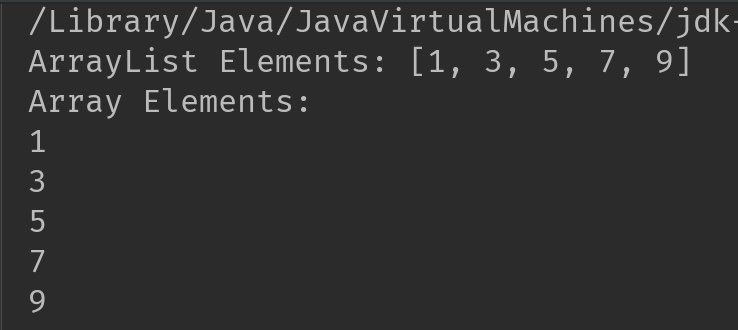