In this example, we will see how to write a java program to split a string by pipe |
symbol.
Java Program to split string by Pipe
Pipe represented by |
symbol, is a meta character in regex. If you simply pass the | in split method like this: str.split("|")
then it would return an unexpected output. Instead of splitting the string by |
, it would create substring for each character in the input string.
To correctly split the string by |
, you need to escape this character and pass this regex in the split method: split("\\|")
Complete Java code:
public class JavaExample{ public static void main(String args[]){ //a string that contains pipe characters String str = "Apple|Orange|Banana|Mango"; //split string by pipe String[] strArray = str.split("\\|"); //print substrings System.out.println("Substring after splitting by pipe: "); for(String s:strArray){ System.out.println(s); } } }
Output:
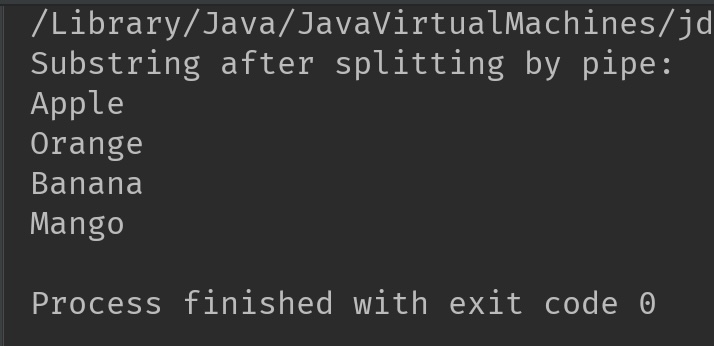
Related Java Guides: