Java Math.floor(double x) method returns the largest integer that is less than or equal to the given argument x
.
public class JavaExample { public static void main(String[] args) { double x = 100.99; //returns a double value equal to an integer //which is less than or equal to x System.out.println(Math.floor(x)); } }
Output:
100.0
Syntax of Math.floor() method
Math.floor(9.001); //returns 9.0
floor() Description
public static double floor(double x): Returns a double value which is less than or equal to x and is equal to a mathematical integer.
floor() Parameters
- x: A double number.
floor() Return Value
- Returns largest double value of the given argument. This value is equal to a mathematical integer and less than or equal to the argument.
- If the argument is NaN, zero or infinity, then it returns the same argument as result.
Example 1: Floor of positive double values
public class JavaExample { public static void main(String[] args) { double x = 0.56; double x2 = 499.100; System.out.println(Math.floor(x)); System.out.println(Math.floor(x2)); } }
Output:

Example 2: Floor of negative double values
public class JavaExample { public static void main(String[] args) { double x = -0.56; double x2 = -499.100; System.out.println(Math.floor(x)); System.out.println(Math.floor(x2)); } }
Output:
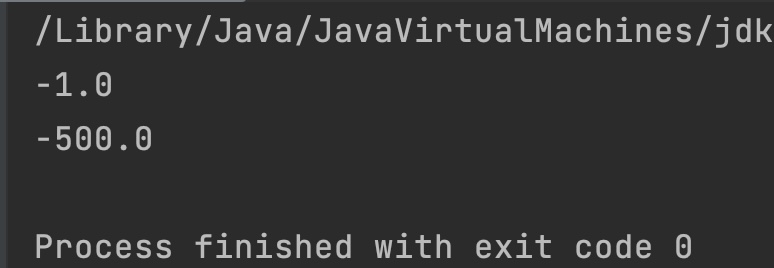
Example 3: Floor value of Double Max and Min Values
public class JavaExample { public static void main(String[] args) { double x = Double.MAX_VALUE; double x2 = Double.MIN_VALUE; System.out.println(Math.floor(x)); System.out.println(Math.floor(x2)); } }
Output:

Example 4: Floor value of positive/negative zero and NaN
public class JavaExample { public static void main(String[] args) { double x = -0.0; //-ve zero double x2 = 0.0; //+ve zero double x3 = 0.0/0; //NaN System.out.println(Math.floor(x)); System.out.println(Math.floor(x2)); System.out.println(Math.floor(x3)); } }
Output:
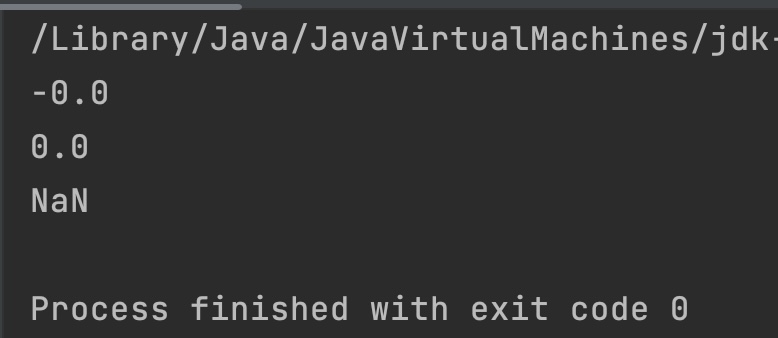