The decode() method decodes a given string into an Integer object. It can accept decimal, hexadecimal and octal strings as argument.
Decimal strings: No prefix such as “102”, “501” etc.
Hexadecimal strings: 0x or 0X prefix such as “0x12E”, “0XEF” etc.
Octal strings: 0 prefix such as 0456, 0357 etc.
Syntax of decode() method
public static Integer decode(String s)
decode() Parameters
s
– The string argument, it represents the string which needs to be decoded into an Integer.
decode() Return Value
- An Integer object that contains the int value represented by string argument s.
Supported java versions: Java 1.2 and onwards.
Example 1: Decode Hexadecimal and Octal strings into Integer
The string prefixed with 0x or 0X are hexadecimal strings. The strings prefixed with 0 are octal strings.
public class JavaExample { public static void main(String[] args) { // no prefix in string is considered decimal value (base 10) System.out.println(Integer.decode("302")); // decode base 16 hexadecimal value 12E to integer // prefix hexadecimal string with 0x or 0X System.out.println(Integer.decode("0x12E")); // decode base 8 octal value 456 to integer // prefix octal string with 0 (zero) System.out.println(Integer.decode("0456")); } }
Output:
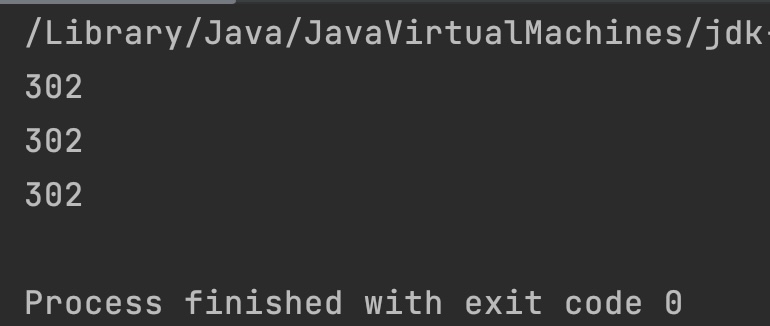
Example 2: Signed strings into Integer
public class JavaExample { public static void main(String[] args) { System.out.println(Integer.decode("-239")); System.out.println(Integer.decode("-0xEF")); System.out.println(Integer.decode("-0357")); } }
Output:
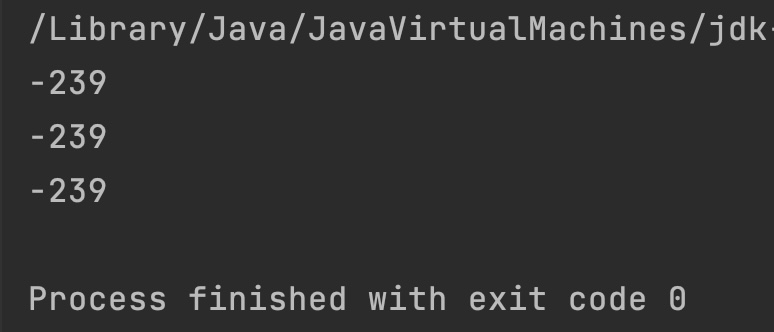
Example 3
public class JavaExample { public static void main(String[] args) { //NumberFormatException if the given string cannot //be decoded into an Integer System.out.println(Integer.decode("0xhello")); } }
Output:
