The hashCode() method returns a hash code for the given integer value.
Syntax of hashCode() method
The following variation of hashCode() method does not accept any argument. It returns the hash code for the int value represented by this Integer object.
Supported versions: Java 1.2 and onwards.
public int hashCode()
An overloaded version of hashCode() method accepts int argument. It returns the hash code for int argument.
Supported versions: Java 1.8 and onwards.
public int hashCode(int val)
hashCode() Parameters
val
– An int value whose hash code is to be determined.
hashCode() Return Value
- A hash code for int value represented by this Integer object or hash code for int argument.
Example 1
public class JavaExample { public static void main(String[] args) { //Integer object contains an int value 101 Integer i = new Integer(101); // hash code for int value 101 int hc = i.hashCode(); System.out.println("Hash code for Integer object: "+hc); int i2 = 151; int hc2 = Integer.hashCode(i2); System.out.println("Hash code for int value 151: "+hc2); } }
Output:
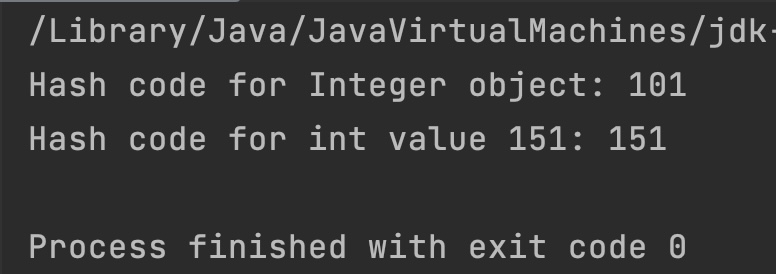
Example 2
import java.util.Scanner; public class JavaExample { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Enter an integer value: "); int i = scan.nextInt(); int hc = Integer.hashCode(i); System.out.println("Hash code for entered value: "+hc); } }
Output:
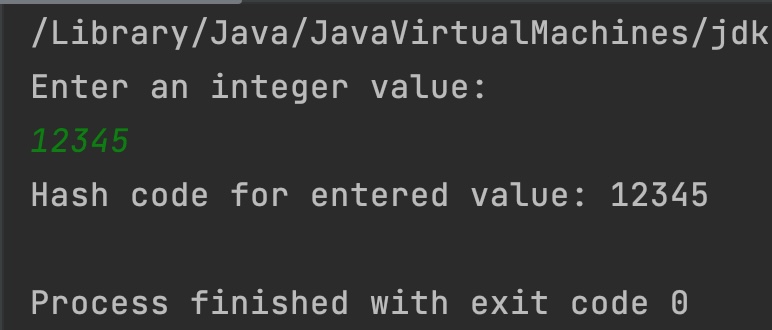
Example 3
public class JavaExample { public static void main(String[] args) { //if an int value is not passed while //creating Integer object. Integer i = new Integer("hello"); System.out.println(i.hashCode()); } }
Output:
