Java Integer equals(Object obj) method compares this Integer object to the given object obj. It returns true if both the objects contain same int value else it returns false.
Hierarchy:
java.lang Package -> Integer Class -> equals() Method
Syntax of equals() method
public boolean equals(Object obj)
equals() Parameters
obj
– The given object to compare with this Integer.
equals() Return Value
- It returns
true
if the objects are same, else it returnsfalse
.
Supported java versions: Java 1.2 and onwards.
Example 1
Comparing three Integer objects with each other.
public class JavaExample { public static void main(String[] args) { Integer i = new Integer(99); Integer i2 = new Integer(35); Integer i3 = new Integer("99"); System.out.print("Integer objects i and i2 are equal: "); System.out.println(i.equals(i2)); System.out.print("Integer objects i and i2 are equal: "); System.out.println(i.equals(i3)); } }
Output:
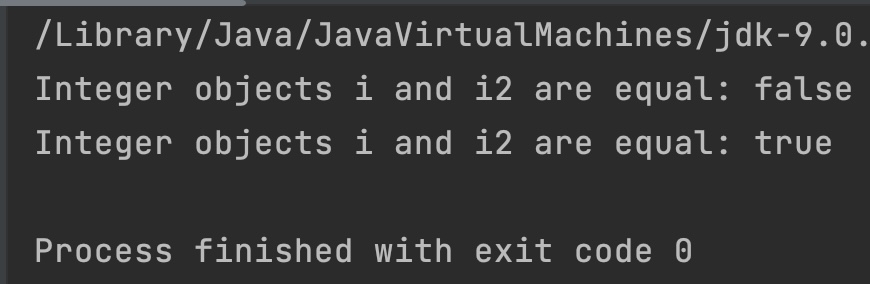
Example 2
Comparing two Integer values entered by user.
import java.util.Scanner; public class JavaExample{ public static void main(String[] args){ Integer i, i2; Scanner scan = new Scanner(System.in); System.out.print("Enter first integer number: "); i = scan.nextInt(); System.out.print("Enter second integer number: "); i2 = scan.nextInt(); if(i.equals(i2)){ System.out.println("Entered numbers are equal"); }else{ System.out.println("Entered numbers are not equal"); } } }
Output:
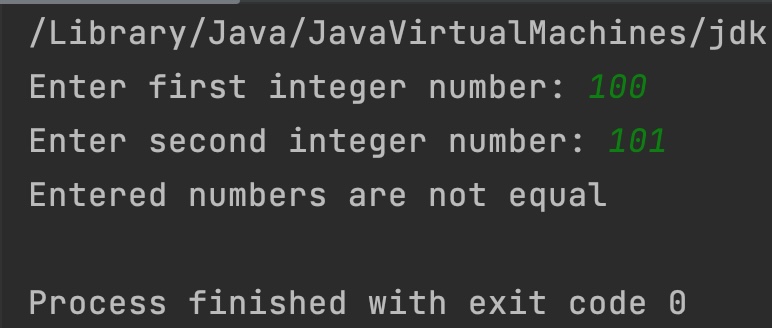
Example 3
Two given strings are decoded using Integer decode() method and compared using equals() method.
public class JavaExample{ public static void main(String[] args){ Integer i, i2; String s1 = "1002"; String s2 = "1002"; i = Integer.decode(s1); i2 = Integer.decode(s2); boolean b = i.equals(i2); System.out.println("Given Strings contain same int value? "+b); } }
Output:
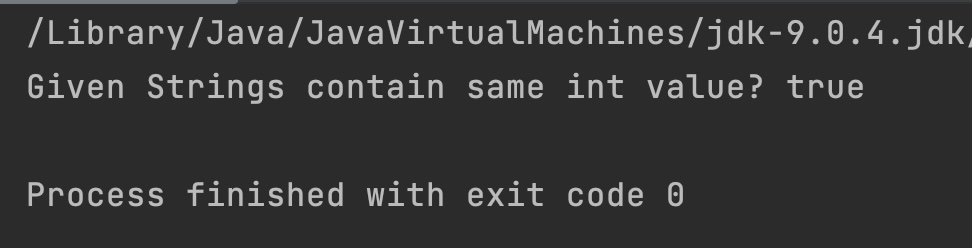