Java StringBuilder codePointCount(int beginIndex, int endIndex) returns the code point count between the specified indexes. In this tutorial, we will discuss codePointCount() method with examples.
Syntax of codePointCount() Method:
int cpCount = sb.codePointCount(2, 5);
The above statement would return the code point count for the characters between index 2 and index 5 in this sequence.
codePointCount() Description
public int codePointCount(int beginIndex, int endIndex): It looks into the range specified by beginIndex
and endIndex
, in the sequence represented by the instance of StringBuilder class. Counts the code points available in the range and returns this count.
codePointCount() Parameters
This method takes two parameters:
- beginIndex: Integer Index value that represents the start of the range.
- endIndex: Integer Index value, it represents the end of the range. This index is exclusive so the character present at this index is not included in the code point count.
codePointCount() Return Value
- Returns the code point counts in the specified range.
It throws IndexOutOfBoundsException
, if any of the following condition occurs:
beginIndex < 0
endIndex > sb.length()
beginIndex > endIndex
Example 1: Number of Unicode code points in whole sequence
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Welcome\uD83D\uDE0A"); //start index first char //end index last char //whole sequence is included in the range int cpCount = sb.codePointCount(0, sb.length()); System.out.println("Sequence is: "+sb); System.out.println("Code Point Count for whole Sequence: "+cpCount); } }
Output:
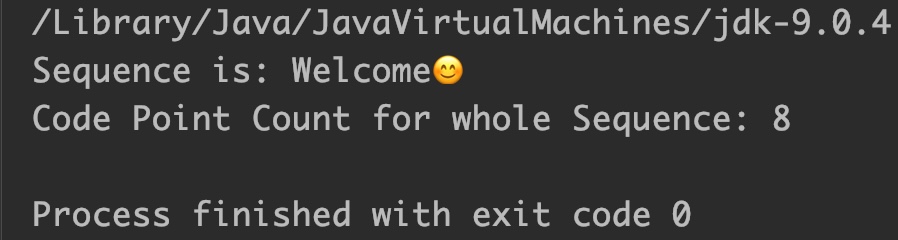
Example 2: Code Point count for a sub sequence
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Welcome\uD83D\uDE0A"); //from index 7 till end int cpCount = sb.codePointCount(7, sb.length()); System.out.println("Sequence is: "+sb); System.out.println("Sub sequence: "+sb.subSequence(7, sb.length())); System.out.println("Code Point Count from index 7 till end: "+cpCount); } }
Output:
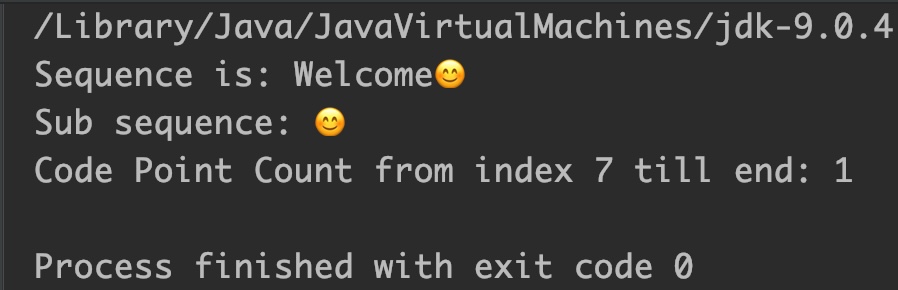
Example 3: If beginIndex > endIndex
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Welcome\uD83D\uDE0A"); //if beginIndex > endIndex int cpCount = sb.codePointCount(7, 6); System.out.println(cpCount); } }
Output:
