Java StringBuilder subSequence() method returns a sub sequence based on the start and end indexes. As we know, StringBuilder is a char sequence, the subSequence() method returns a subset of this sequence.
Syntax of subSequence() Method:
//returns the char sequence from index 2 to 5 //2 is inclusive and 5 is exclusive CharSequence cs = sb.subSequence(2, 5);
Here, sb is an object of StringBuilder class.
subSequence() Description
public CharSequence subSequence(int start, int end): It returns a subsequence of the current StringBuilder sequence based on the indexes.
subSequence() Parameters
This method takes two parameters:
- start: It is an integer index that represents the start of the subsequence. It is inclusive.
- end: Integer index that represents the end of the subsequence. It is exclusive
subSequence() Return Value
- It returns a new char sequence which is a subset of the old char sequence.
It throws IndexOutOfBoundsException
, if any of the following condition occurs:
start < 0
orend <0
end >= sb.length()
start > end
Example 1: Get a subsequence from StringBuilder instance
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Welcome"); System.out.println("Sequence: "+sb); //getting sub sequence from index 3 to 7 CharSequence cs = sb.subSequence(3, 7); System.out.println("Sub sequence: "+cs); } }
Output:
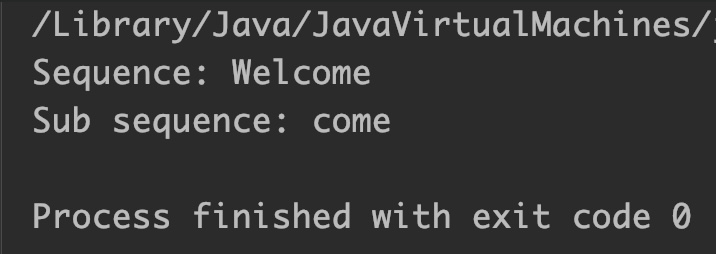
Example 2: Remove first and last character from Sequence
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Home"); System.out.println("Given Sequence: "+sb); //from 2nd char till 2nd last char CharSequence cs = sb.subSequence(1, sb.length()-1); System.out.println("Sub sequence: "+cs); } }
Output:
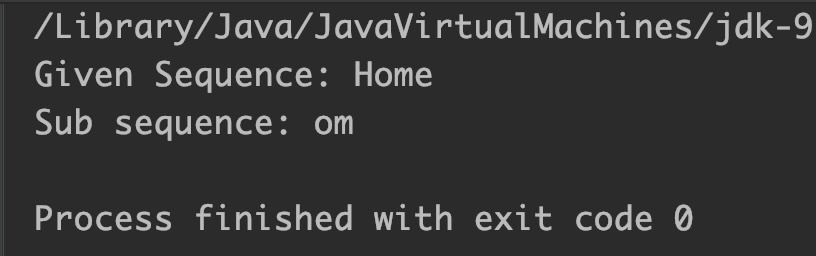
Example 3: start Index > end Index
public class JavaExample { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Home"); System.out.println("Given Sequence: "+sb); //if start index > end index CharSequence cs = sb.subSequence(2, 1); System.out.println("Sub sequence: "+cs); } }
Output:
